Instantly share code, notes, and snippets.

gitaficionado / Assignment2_Demo.java
- Download ZIP
- Star ( 0 ) 0 You must be signed in to star a gist
- Fork ( 0 ) 0 You must be signed in to fork a gist
- Embed Embed this gist in your website.
- Share Copy sharable link for this gist.
- Clone via HTTPS Clone using the web URL.
- Learn more about clone URLs
- Save gitaficionado/31564adf3b1ad5db042364d6151bc710 to your computer and use it in GitHub Desktop.
/* Assignment 2 - Control Tower */ | |
/* Class name - must be "Assignment2" in order to run*/ | |
import java.util.Scanner; | |
//import edhesive.assignment2.Airplane; | |
public class Assignment2 | |
{ | |
public static void main(String[] args) | |
{ | |
// Create Scanner and Airplane 1 | |
______________ scan = __________ Scanner(System.in); // Create Scanner object here. | |
Airplane ___ = new _______________(); //create new object of type Airplane called p1 | |
// Get Airplane 2 details | |
System.out.println("Enter the details of the second airplane (call-sign, distance, bearing and altitude):"); | |
String ____g__ = _____.______(); // create string called "cs" for call sign for code sign | |
double dist = scan.nextDouble(); ed | |
____ dir = scan.nextInt(); // Creaete input from user to hold direction. | |
______ _____ = _____.nextInt(); //Creaete input from user to altitude | |
cs = ______.________(); // Use String method to conert call sign to Upper Calse Letters | |
/* To create a plane with the characteristics described above, call | |
* the constructor with the variables matching the signature: | |
* (String cs, double dist, int dir, int alt). | |
*/ | |
_______ _________ = _______ ________(____, ____, ____ , ____ ); // Create new object that takes in call sign | |
// distance, direction, and alt | |
/* When the airplane objects p1 and p2 are concatenated into a | |
* String, the toString method is called automatically, causing the | |
* representation requested by the assignment to be printed. When | |
* the method call p1.distTo(p2) is concatenated into the String, | |
* the result of this call is concatenated into the string. | |
*/ | |
System.out.println("\nInitial Positions:"); | |
System.out.println("\"Airplane 1\": " + ___); | |
System.out.println("\"Airplane 2\": " + ___); | |
System.out.println("The distance between the planes is " + ___.______(p2) + " _____."); | |
System.out.println("The difference in height between the planes is " + ______ .____ (___ __() - ___ _____()) + " feet."); | |
// Calling the gainAlt method 4 times moves the plane up 4000 feet | |
p1.____________(); | |
p1.___________(); | |
p1.___________(); | |
p1.__________(); | |
//Calling the loseAlt method 2 times moves the plane down 2000 feet | |
___.________(); | |
___.___________(); | |
// Moves the planes the desired distances and directions | |
___.____________(10.5, 50); | |
__.move(8.0, 125); | |
//Repeat print statements from before | |
System.out.println("\nNew Positions:"); | |
System.out.println("\"Airplane 1\": " + __); | |
System.out.println("\"Airplane 2\": " + ___); | |
System.out.println("The distance between the planes is " + ___.________(p2) + " miles."); | |
System.out.println("The difference in height between the planes is " + ________________)-___.________()) + " feet."); | |
} | |
} |

# Unit 2: Using Objects
# lesson 1: strings and class types, # lesson 2: escape sequences and string concatenation, # lesson 3: string methods, # lesson 4: classes and objects, # lesson 5: using constructors, # lesson 6: using methods, # lesson 7: wrapper classes, # lesson 8: math functions, # assignment 2: control tower.
- For educators
- English (US)
- English (India)
- English (UK)
- Greek Alphabet
This problem has been solved!
You'll get a detailed solution from a subject matter expert that helps you learn core concepts.
Question: JAVA In this assignment, you will be simulating an Air Traffic Control tower. This program uses data of the Airplane class type. This is a custom class that you will use for this activity. Each Airplane object represents an actual airplane that is detected by the tower at a particular instance in time. The Airplane object has a number of fields: a horizontal
JAVA In this assignment, you will be simulating an Air Traffic Control tower. This program uses data of the Airplane class type. This is a custom class that you will use for this activity. Each Airplane object represents an actual airplane that is detected by the tower at a particular instance in time. The Airplane object has a number of fields: a horizontal distance in miles from the tower (as a positive decimal number), a bearing (compass direction) from the tower (as an integer from 0 to 360), a positive altitude (height) in feet (as a positive integer) and a call-sign which consists of letters, numbers, and symbols. The Airplane class has the following constructors and methods: Constructors Airplane() - Creates an Airplane with call sign “AAA01” located on the landing strip: 1 mile due north (0°) of the tower at an altitude of 0 feet. Airplane(String cs, double dist, int dir, int alt) - Creates an Airplane with call-sign cs dist miles from the tower on a bearing of dir degrees, at an altitude of alt feet. Notes: alt and dist will always be read as absolute values (non-negative). If dir is not between 0 and 360, the bearing will be set to dir % 360. Methods move(double dist, int dir) - Void method. Changes the Airplane position by dist miles on a heading of dir degrees. gainAlt() - Void method. Increases the altitude of the Airplane by 1000 feet. loseAlt() - Void method. Decreases the altitude of the Airplane by 1000 feet, or to 0 if altitude is less than 1000 feet. getAlt() - Returns an int representing the altitude of the Airplane. toString() - Returns a String representation of the Airplane including all fields. For example: AAL123 - 110.5 miles away at bearing 059°, altitude 4500 feet distTo(Airplane other) - Returns a double representing the distance in miles between this Airplane and the Airplane other You will first write code to create a plane, "Airplane 1", with the default callsign of AAA01, starting in the default position of 1 mile due north (0°) of the tower at an altitude of 0 feet. Your program will then create a second plane, “Airplane 2”, with the callsign of AAA02, starting at a position of 15.8 miles due north (128°) of the tower at an altitude of 30,000 feet. Next, your program should ask the user to input the details of a third airplane, "Airplane 3", detected by the tower. This will consist of the call-sign, distance, direction and altitude. Once these inputs have been entered, your program should convert the callsign to use uppercase letters, then create Airplane 3 using these details. Now, your program should make the following changes to the positions of the airplanes: Increase the altitude of Airplane 1 by 3,000 feet. Decrease the altitude of Airplane 2 by 2,000 feet. Decrease the altitude of Airplane 3 by 4,000 feet. Move Airplane 1 a distance that is equal to the initial distance between Airplane 2 and Airplane 3 on a heading of 65°. In other words, if the distance between Airplane 2 and Airplane 3 is 4.6 miles, then we should move Airplane 4.6 miles on a heading of 65°. Move Airplane 2 a distance of 8.0 miles on a heading of 135°. Move Airplane 3 a distance of 5.0 miles on a heading 55°. After this, your program should print the details of the planes with their new positions, the new distances between each of the airplanes, and the new differences in height between each of the airplanes. You should carefully follow the format shown below in the sample runs when you create your program: make sure your program produces the exact same output when you input the sample data into it. Milestones Milestone 1: Write a constructor call to create the first default plane. Write a constructor call to create a second plane with the non-default values. Then write code to get inputs of the correct type for each field for the third plane. Convert the callsign to uppercase and use this data to create Airplane 3. Milestone 2: Write code that prints the relevant details for all three airplanes. Calculates the distance between each of the airplanes and the (positive) difference in altitude between each of the airplanes, then prints these values. Milestone 3: Write code that moves the planes as desired (the first airplane up 3000 feet, the second down 2000 feet, and the third down 4,000 feet, then move the first airplane by the initial distance between the second and third on a heading of 65°, the second 8.0 miles on a heading of 135°, and the third 5.0 miles on a heading of 55°). Repeat the code from milestone 2 to print the new positions. Sample Runs Sample Run 1 Enter the details of the third airplane (call-sign, distance, bearing and altitude): UaL256 12.8 200 22000 Initial Positions: "Airplane 1": AAA01 - 1.0 miles away at bearing 000°, altitude 0 feet "Airplane 2": AAA02 - 15.8 miles away at bearing 128°, altitude 30000 feet "Airplane 3": UAL256 - 12.8 miles away at bearing 200°, altitude 22000 feet Initial Distances: The distance between Airplane 1 and Airplane 2 is 16.43 miles. The distance between Airplane 1 and Airplane 3 is 13.74 miles. The distance between Airplane 2 and Airplane 3 is 16.98 miles. Initial Height Differences: The difference in height between Airplane 1 and Airplane 2 is 30000 feet. The difference in height between Airplane 1 and Airplane 3 is 22000 feet. The difference in height between Airplane 2 and Airplane 3 is 8000 feet. New Positions: "Airplane 1": AAA01 - 17.43 miles away at bearing 062°, altitude 3000 feet "Airplane 2": AAA02 - 23.76 miles away at bearing 130°, altitude 28000 feet "Airplane 3": UAL256 - 9.16 miles away at bearing 182°, altitude 18000 feet New Distances: The distance between Airplane 1 and Airplane 2 is 23.62 miles. The distance between Airplane 1 and Airplane 3 is 23.4 miles. The distance between Airplane 2 and Airplane 3 is 19.5 miles. New Height Differences: The difference in height between Airplane 1 and Airplane 2 is 25000 feet. The difference in height between Airplane 1 and Airplane 3 is 15000 feet. The difference in height between Airplane 2 and Airplane 3 is 10000 feet.
this code is by done in java language with 2 steps.

Not the question you’re looking for?
Post any question and get expert help quickly.
Control Tower #
A Control Tower is an advanced data collection, integration and standardization tool for operations management. The technology offers end-to-end visibility of operations in real-time, enabling very realistic decision making. The Control Tower is intended to consolidate data from internal and external business partners. This strategy provides real-time visibility into supply chain operations and enables better informed decision-making based on a holistic picture of the facts. Control Towers can be combined with Digital Process Automation to create a fully digitalized process control environment. They can be applied to many stages of operations management, such as transportation, warehousing, production, co-packing and even a global end-to-end perspective.
The Control Tower predicts and anticipates probable interruptions with the use of machine learning algorithms, allowing for proactive response and exception management. The Control Tower provides various advantages, such as greater awareness and insight into supply chain operations, the ability to address possible interruptions and the capacity to execute and fulfill supply chain objectives through a collaborative intelligent response framework. Through the capturing of particulars and specified empirical values, the Control Tower also facilitates ongoing discovery and learning.
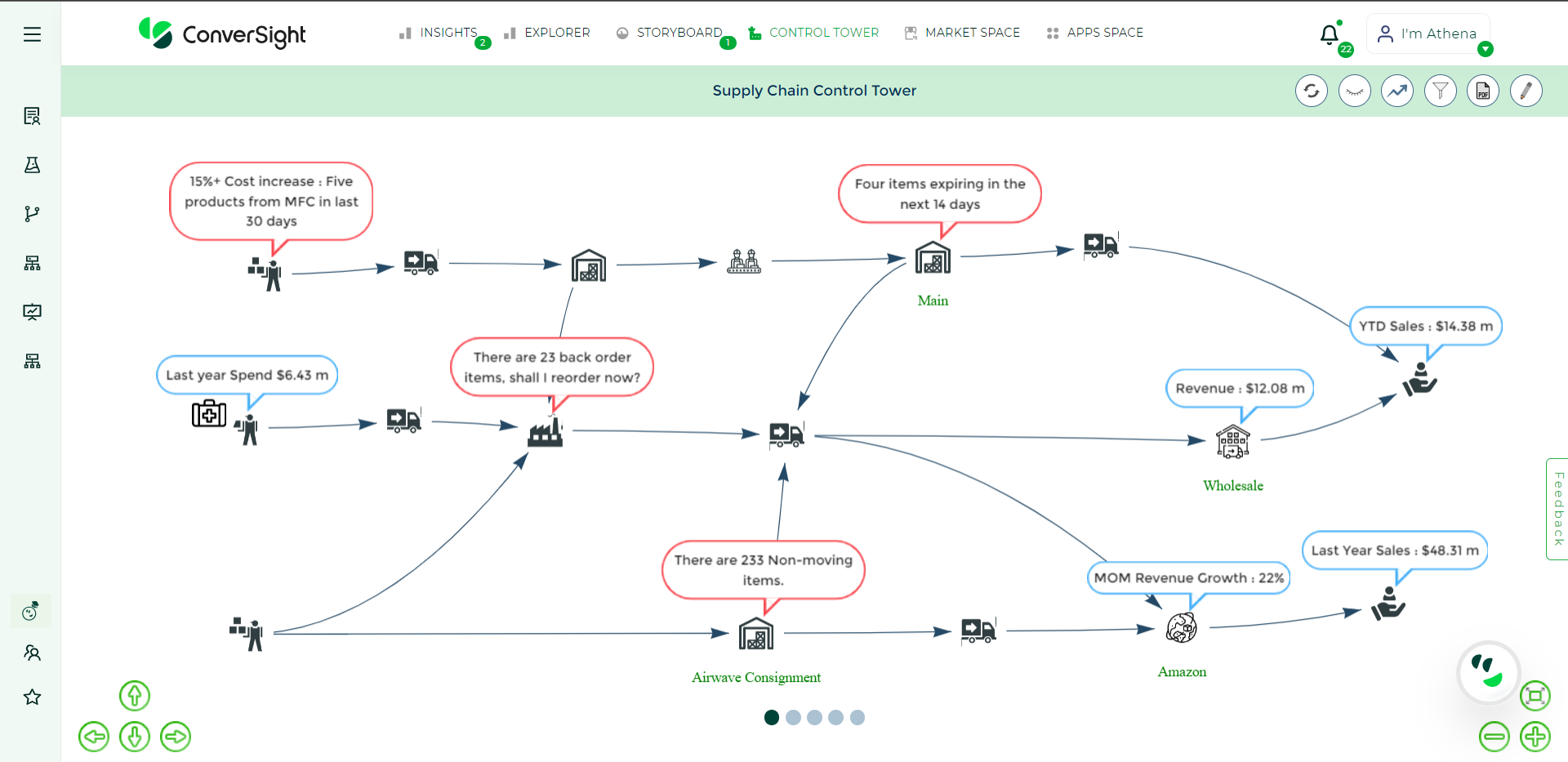
The data acquired by the Control Tower is standardized and provides a source of personalized information, providing for comprehensive supply chain visibility. This enables the company’s various divisions and areas, as well as its external partners, to collaborate in attaining the operations worldwide objectives.
Control Tower offer a comprehensive solution for managing the complexities of the supply chain. With real-time visibility and advanced analytics, companies can make informed decisions, respond to disruptions proactively and optimize their operations for maximum efficiency and success.
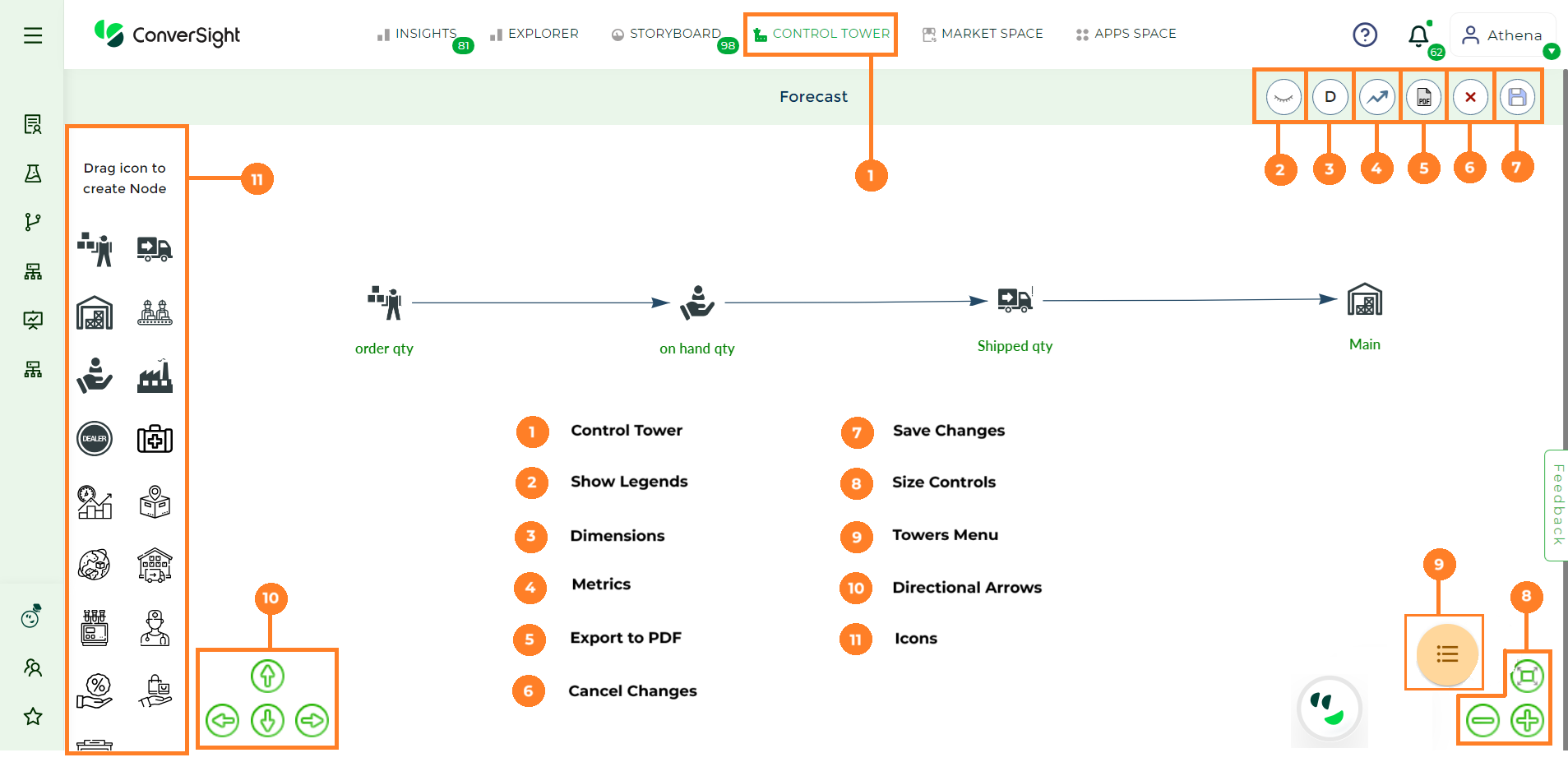
Control Tower Illustration #
To watch the video, click on Control Tower Overview .

Creation of Control Tower #
Let’s explore the process involved in building a Control Tower.
Step 1: Navigate to the Control Tower tab in the landing page.
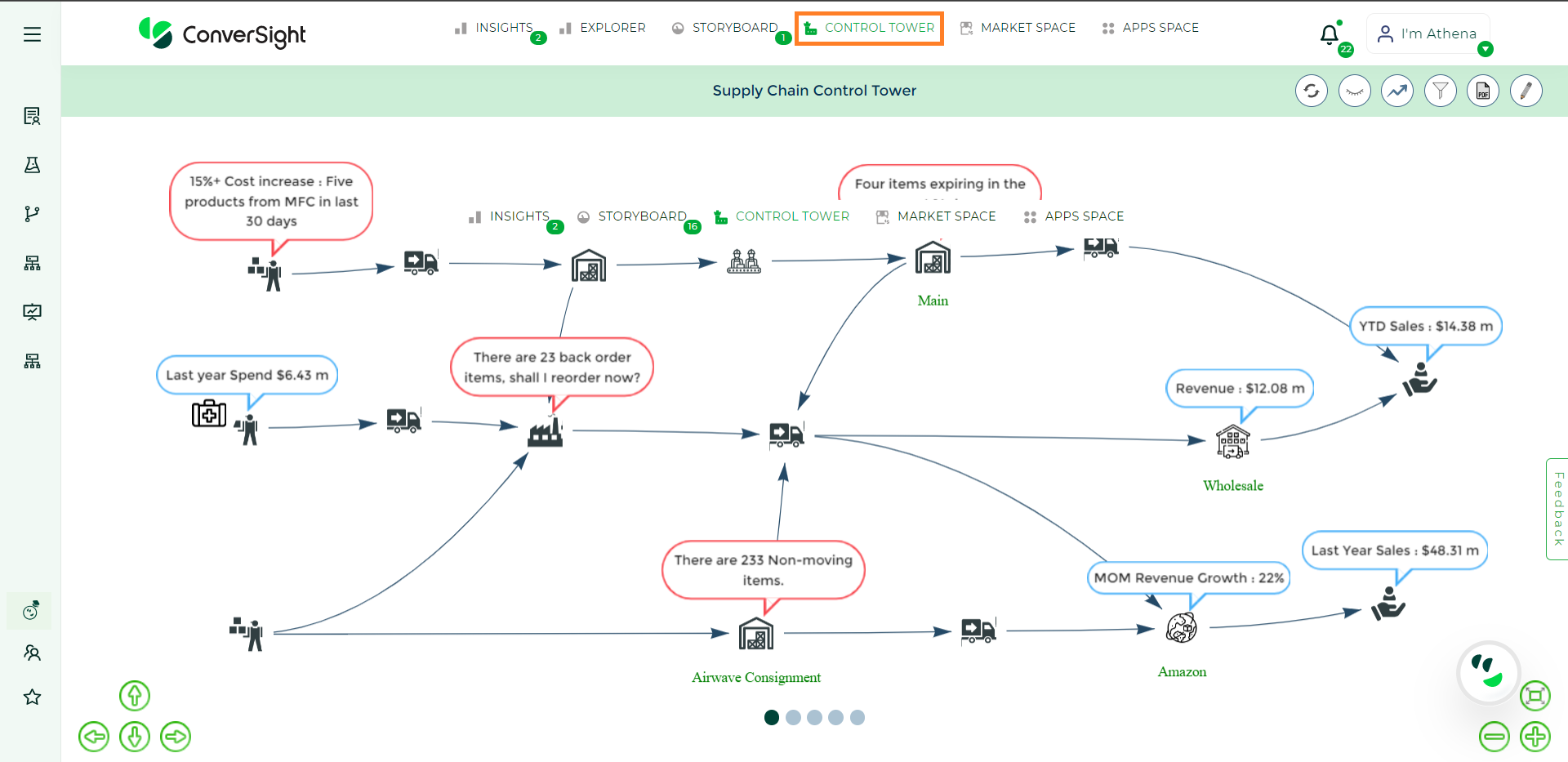
Control Tower Tab #
Step 2: On the Control Tower page, you will encounter several choices. To initiate the creation of a new Control Tower, simply select the Edit icon located on the right-hand side.
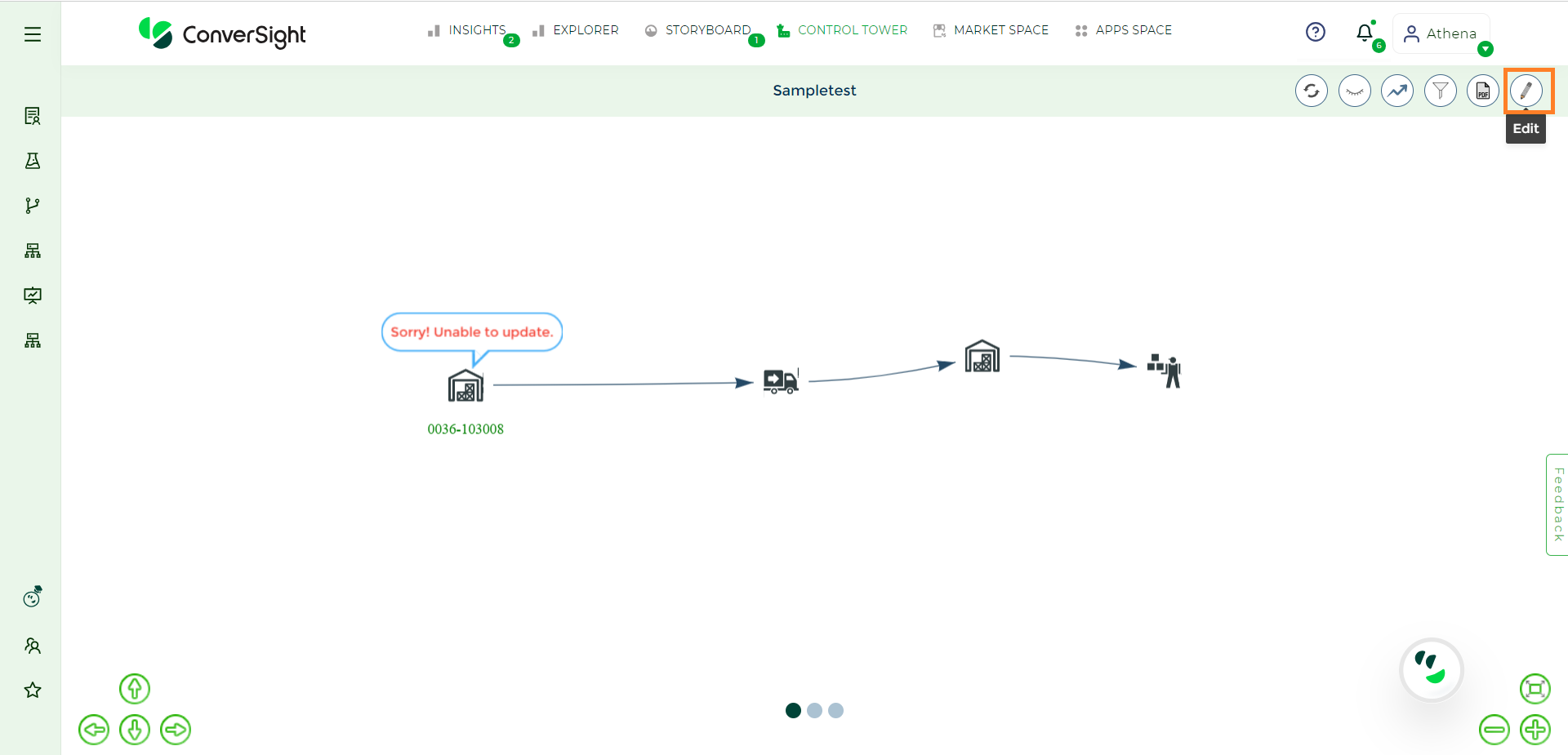
Step 3: Click on the Towers menu to create a new Control Tower.
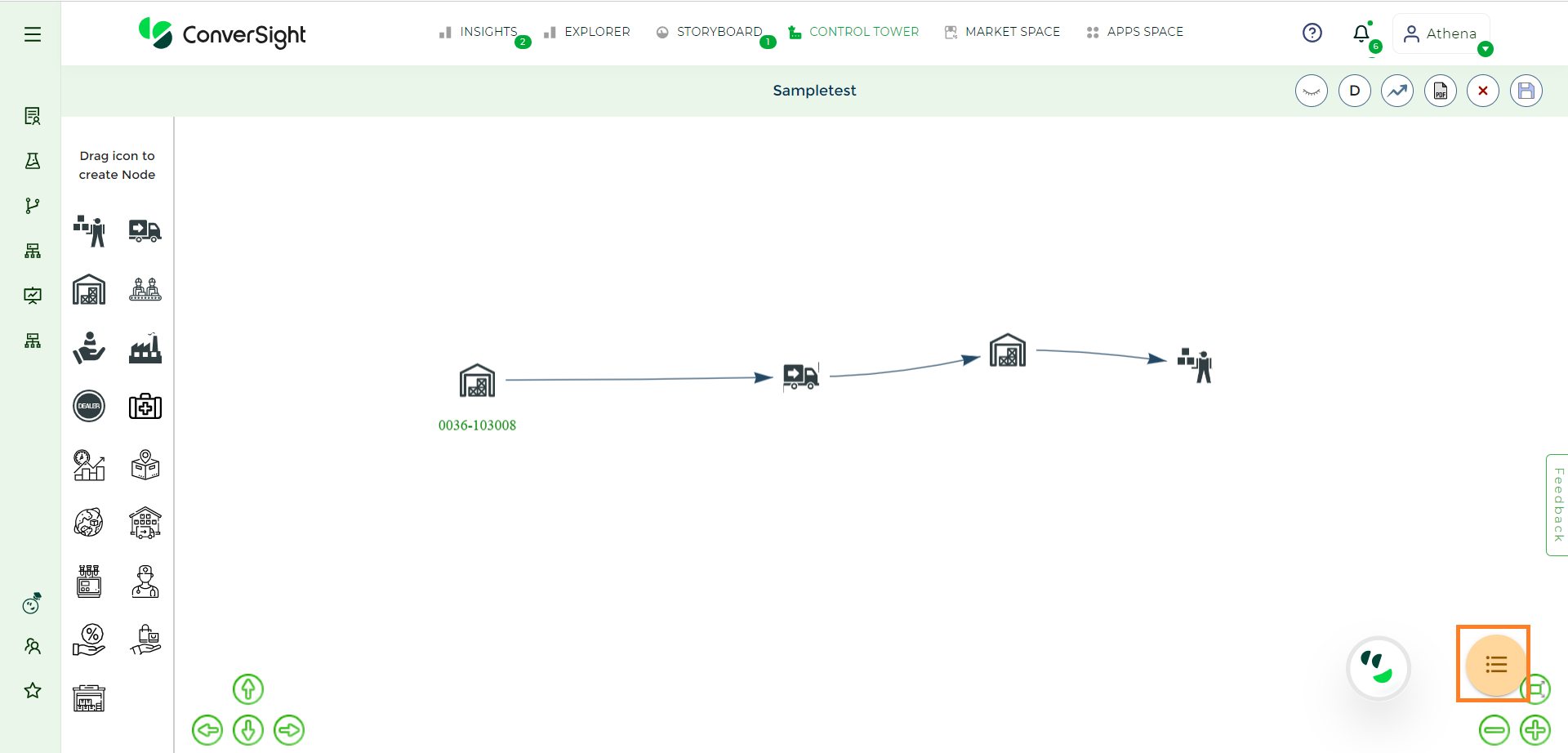
Towers Menu #
Step 4: In the Towers menu, you’ll find a list of Control Towers that have been created. To initiate the creation of a new one, just click on the Add button. Input a name for your Control Tower and proceed to select a dataset.
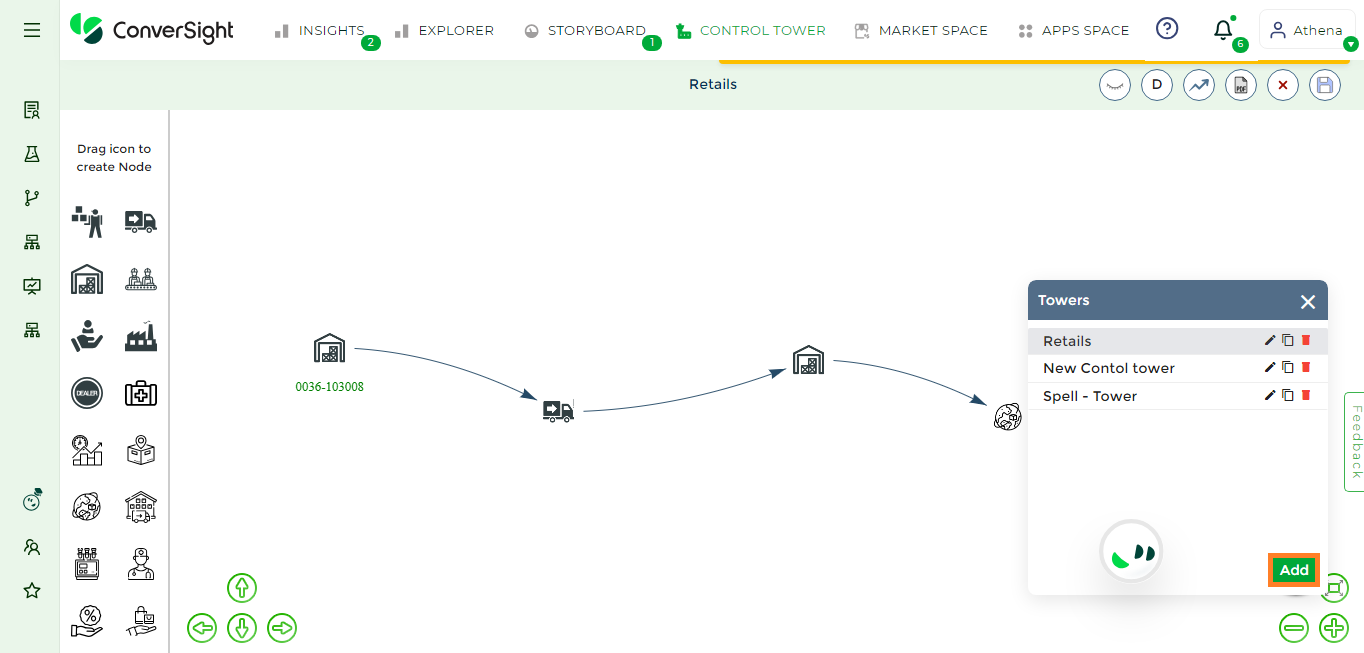
Creating Control Tower #
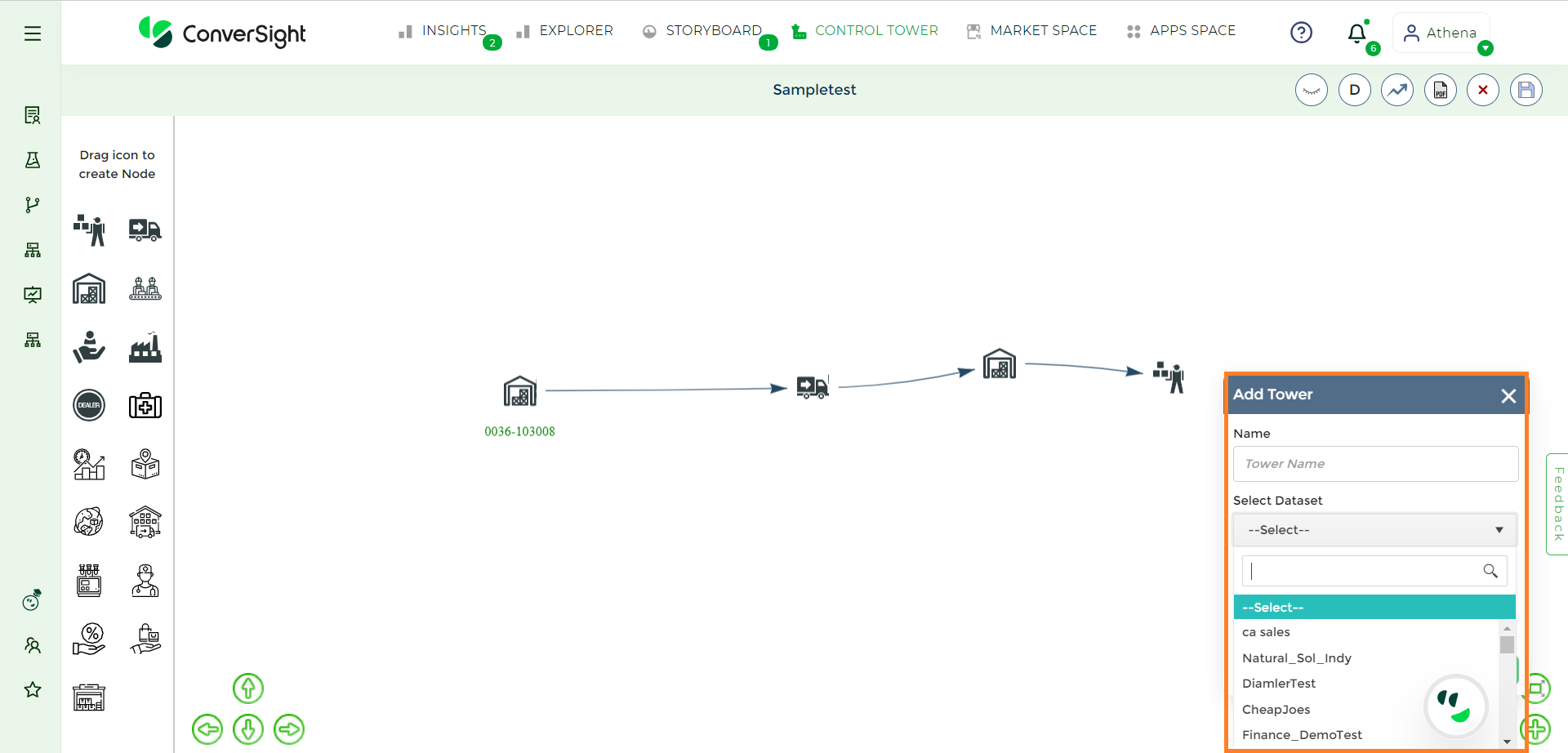
Step 5: Once the name and dataset information has been entered, proceed by clicking the Save button.
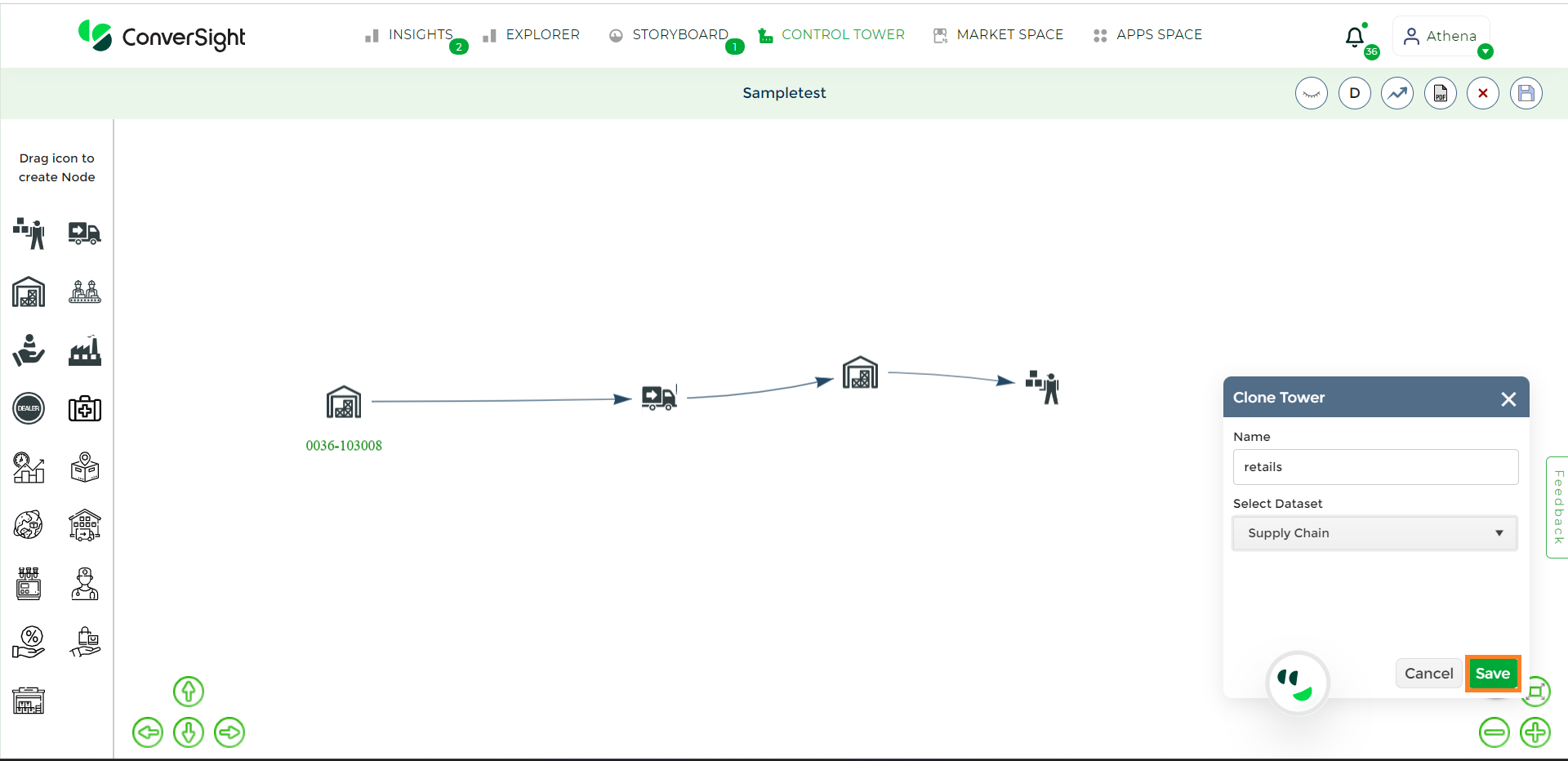
Saving Control Tower #
Step 6: Once the saving process is complete, a blank screen will be presented. Users can then utilize a drag-and-drop approach to construct the Control Tower, enhancing clarity by placing icons that represent their business.
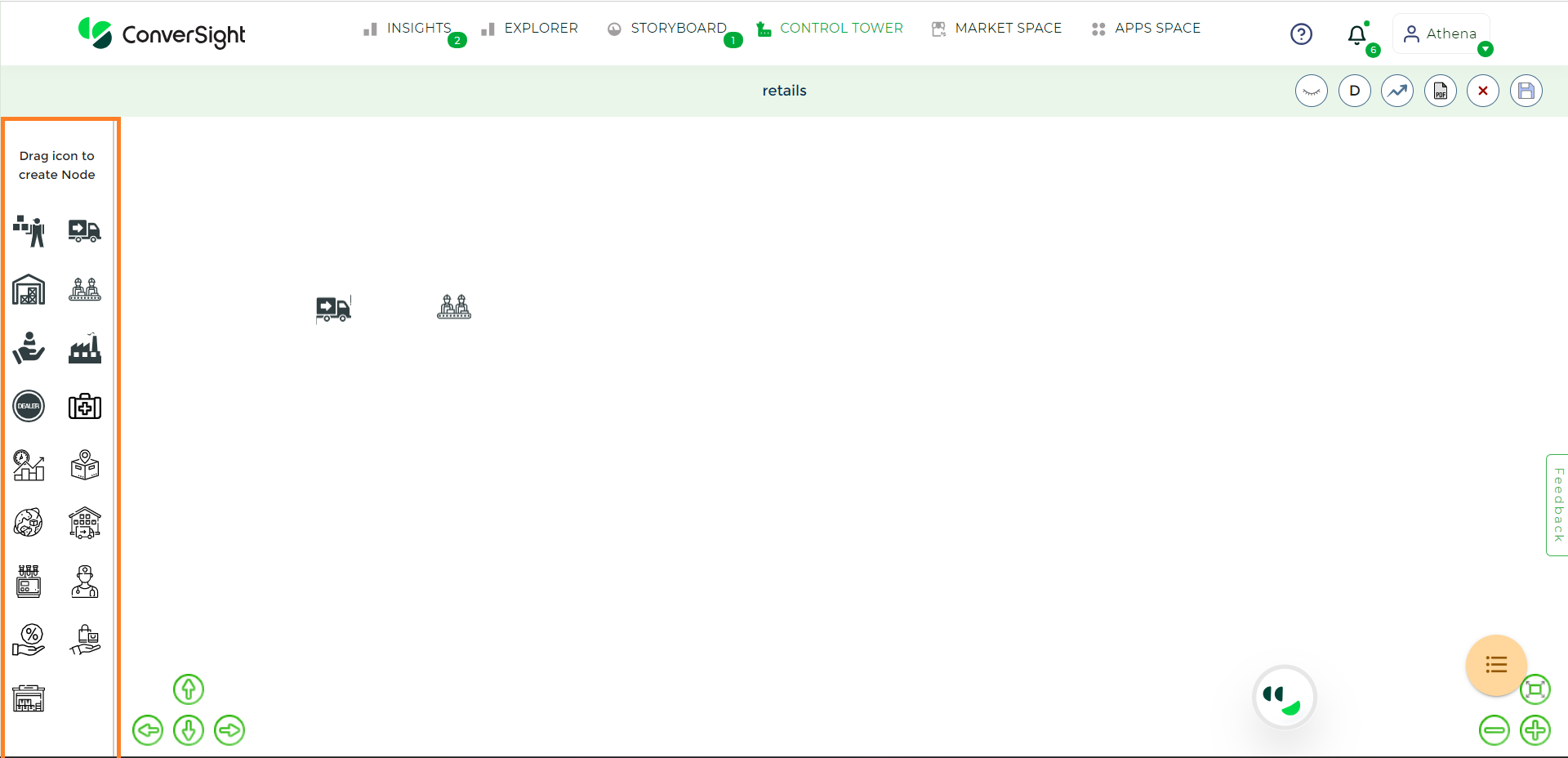
Nodes for building Control Tower #
Step 7: To establish connections between nodes, you can introduce edges. To include edges, just perform a right-click on a node and select the Add Edge choice. This facilitates the linkage between the nodes.
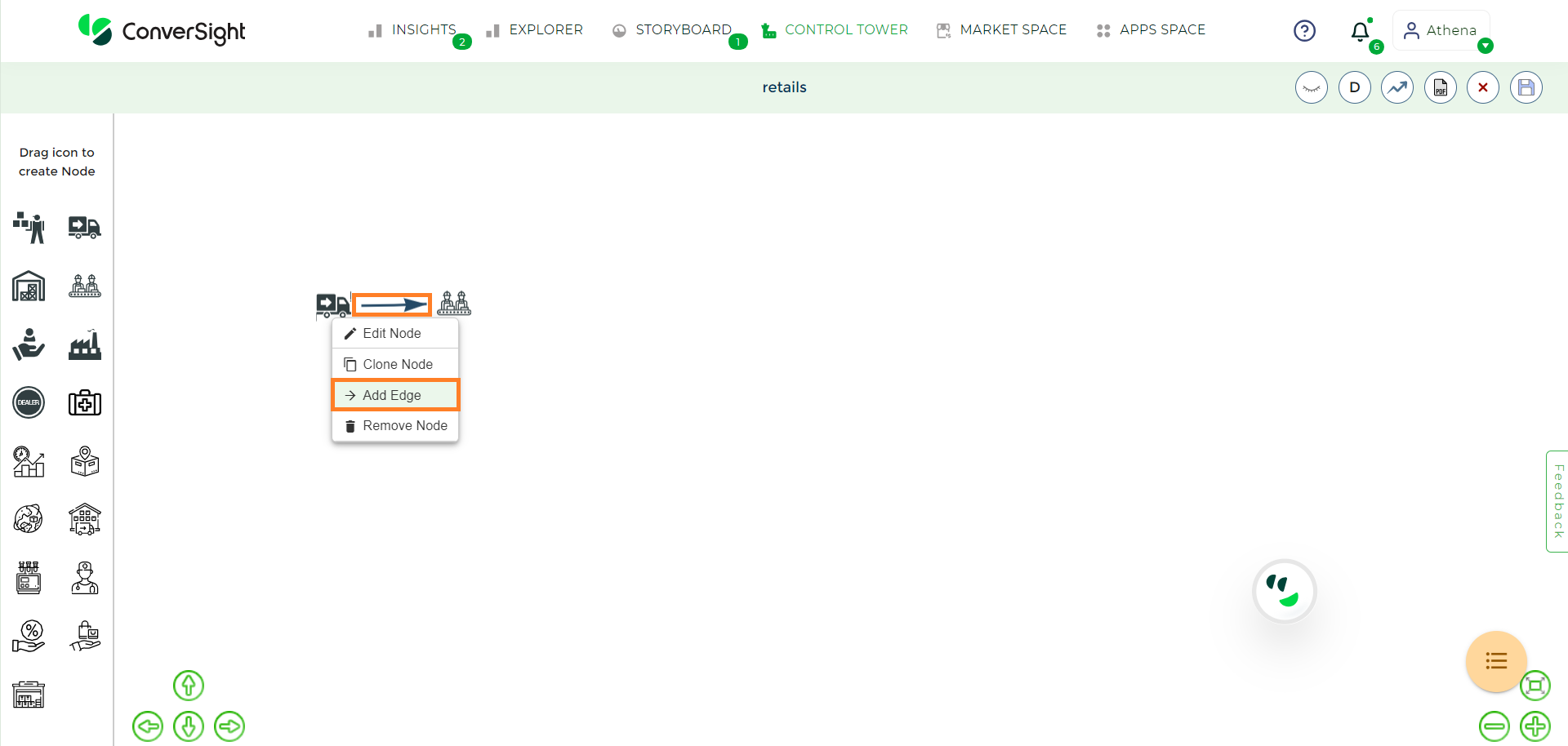
Adding edges in Control Tower #
The steps outlined above provide a foundational guide for establishing a Control Tower. For customizing nodes to match your unique requirements, the following section details the process of editing nodes accordingly.
To watch the video, click on Creating Control Tower .
Edit Node #
The Edit Node feature serves the purpose of personalizing the node and its attributes to align with your requirements. Now, let’s delve into the procedure of node customization.
You can edit the properties of the node by right clicking the node and choosing the Edit Node option.
Editing Node #
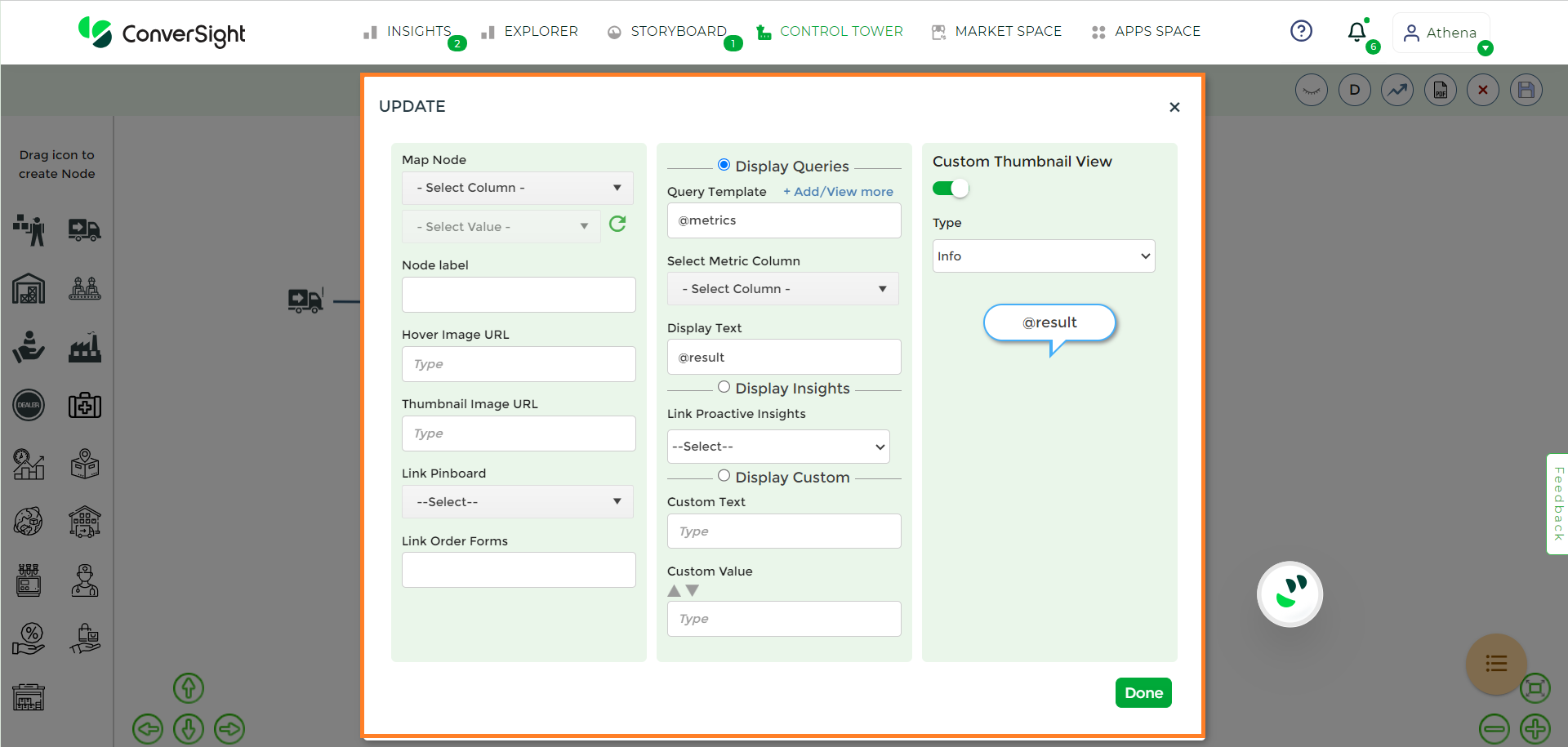
Editing Node properties #
This feature provides a comprehensive view, highlighting crucial information pertaining to each node. The table includes the following columns.
|
|
---|---|
Map Node | You possess the ability to select both the column and its corresponding value from your dataset. |
Node Label | You are presented with the option to input a label or alternatively, the chosen value from the column will be exhibited. |
Hover Image URL | You have the capability to modify the image while in hover state by providing the URL. |
Thumbnail Image URL | To modify the thumbnail image of a node, you can input the respective new URL. |
Link Pinboard | You can connect to your node using the relevant storyboards you’ve generated, which will align with the items you have available. |
Link Order Forms | You can connect your node to Forms in ConverSight based on your requirements. |
NOTE You have the option to associate your node with either a Storyboard or a Form, but it's not possible to connect it to both at the same time.
Display Queries #
In the Display Query section, users have the ability to personalize the query, as well as select the column table value that should appear above the node.
|
|
---|---|
| By default, the query template displays the categories of metrics. |
| You have the option to select the metric column from the chosen dataset. |
| Enter the desired text for display. |
To include multiple queries within the node, click on the + Add/View more option, you can modify your created query and incorporate additional templates that align with your business needs.
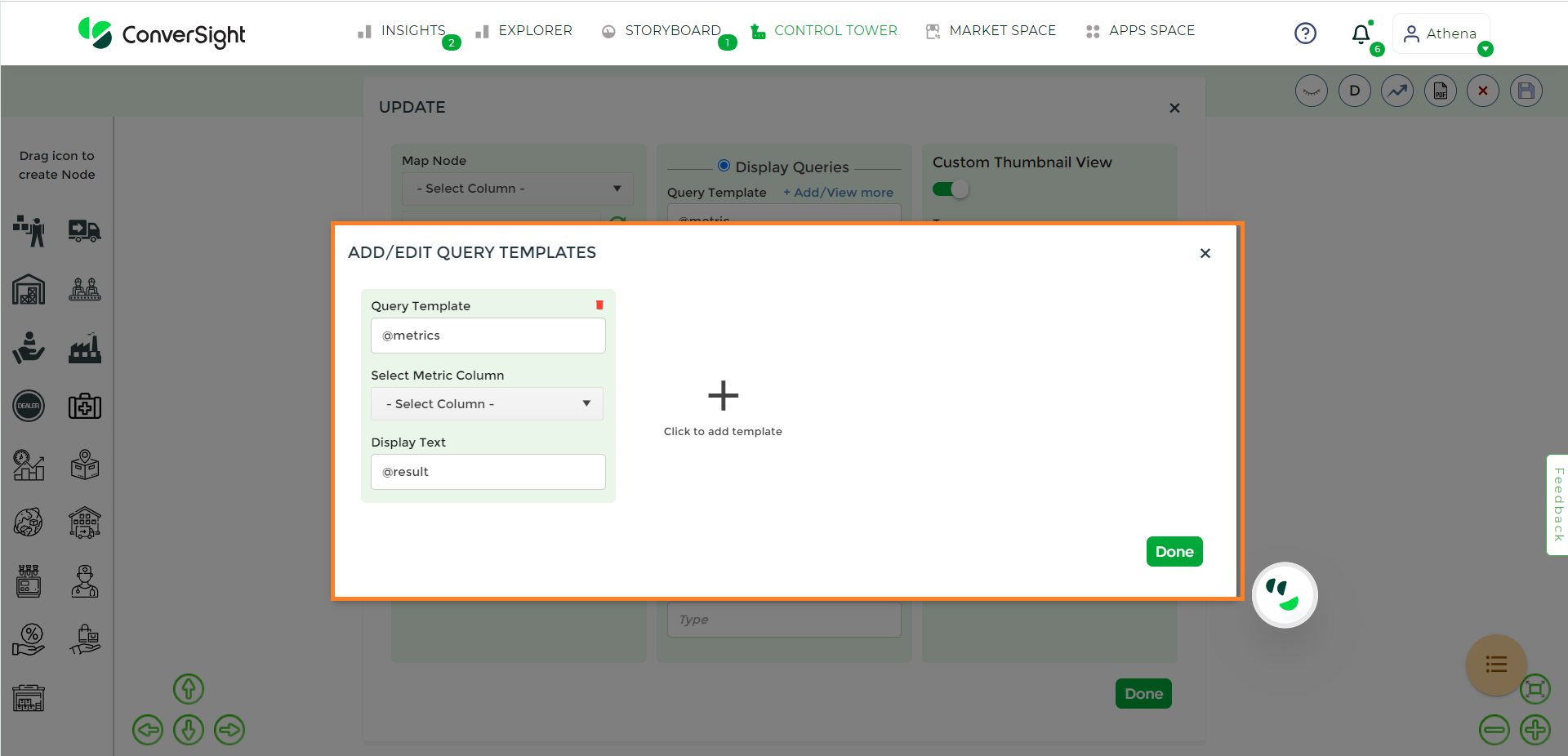
Add/Edit query templates #
Display Insights #
By selecting the Link Proactive Insights option, you can establish a connection between the node and the insights you’ve generated, ensuring alignment with your specific need.
Display Custom #
Opting for the Display Custom feature enables you to include your own text and specified values for showcasing. These custom elements remain unchanged for the selected node and can be manually modified if required.
|
|
---|---|
| You have the option to customize the displayed text according to your preferences. This personalized text remains constant and doesn’t change. |
| You can input the value and additionally, the value can also be indicated with increment or decrement symbol. |
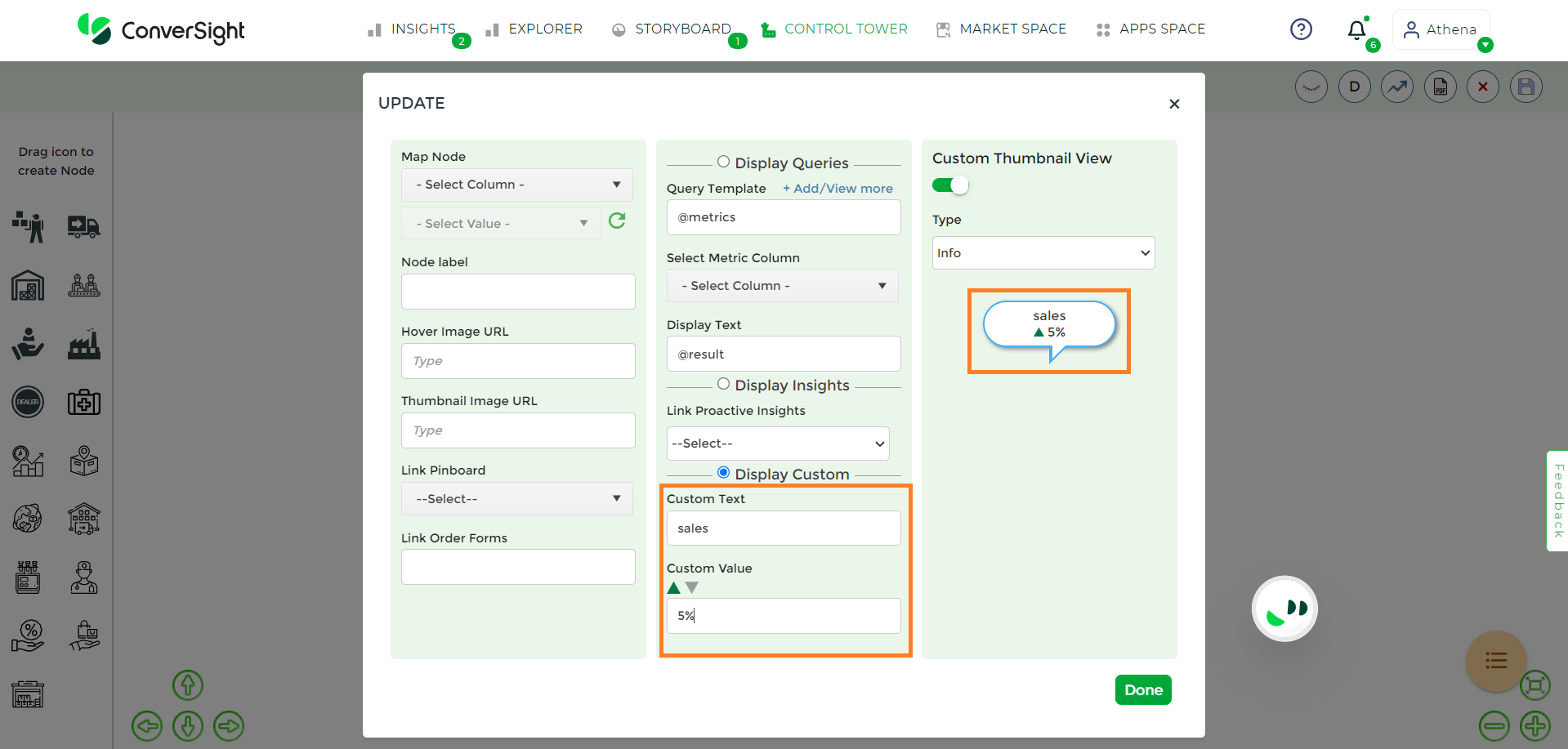
The Type option primarily pertains to the color in which the display is presented. It provides you with the following:
Info: Selecting the info type will result in its display in blue color.
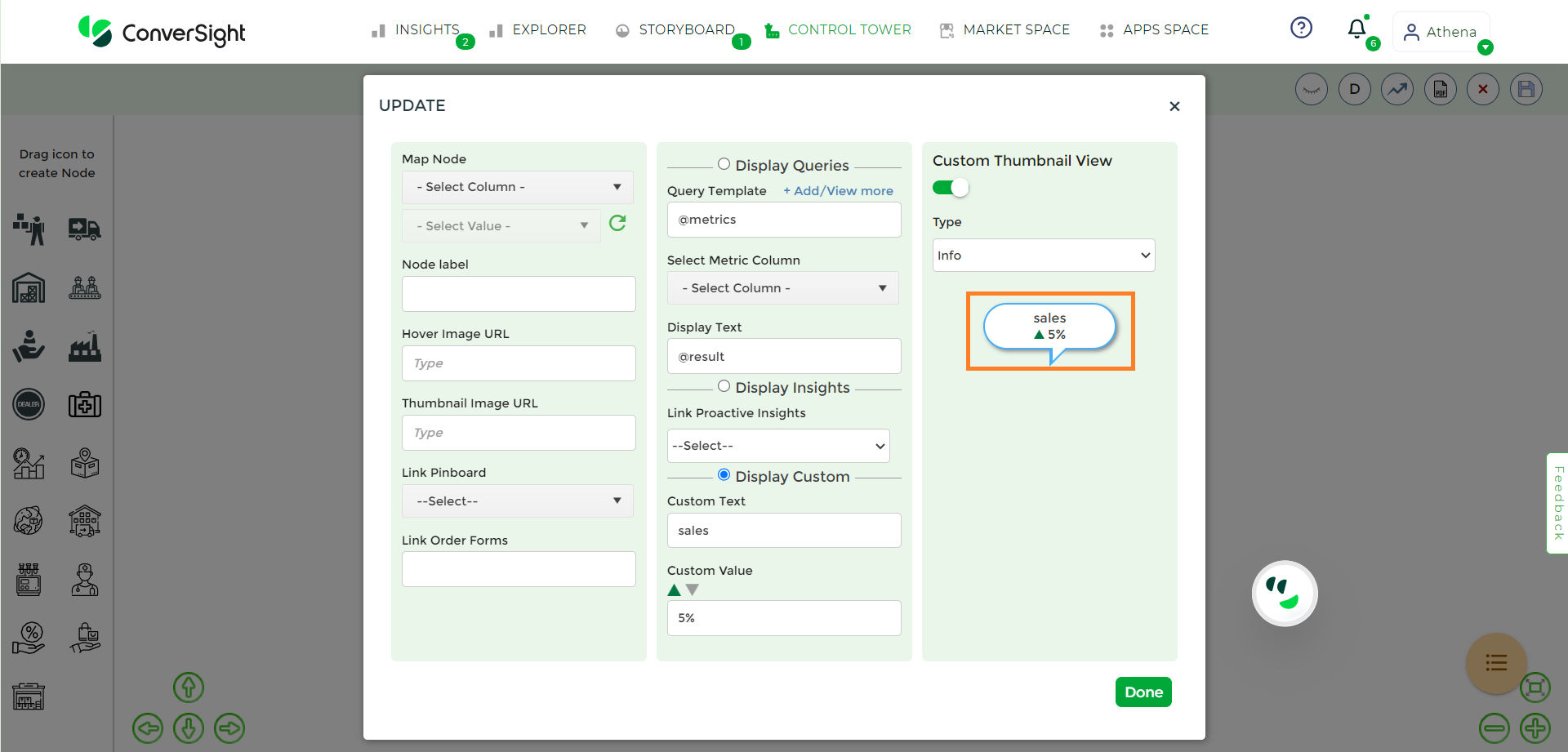
Type Info #
Warning: Selecting the warning type will result in its display in yellow color.
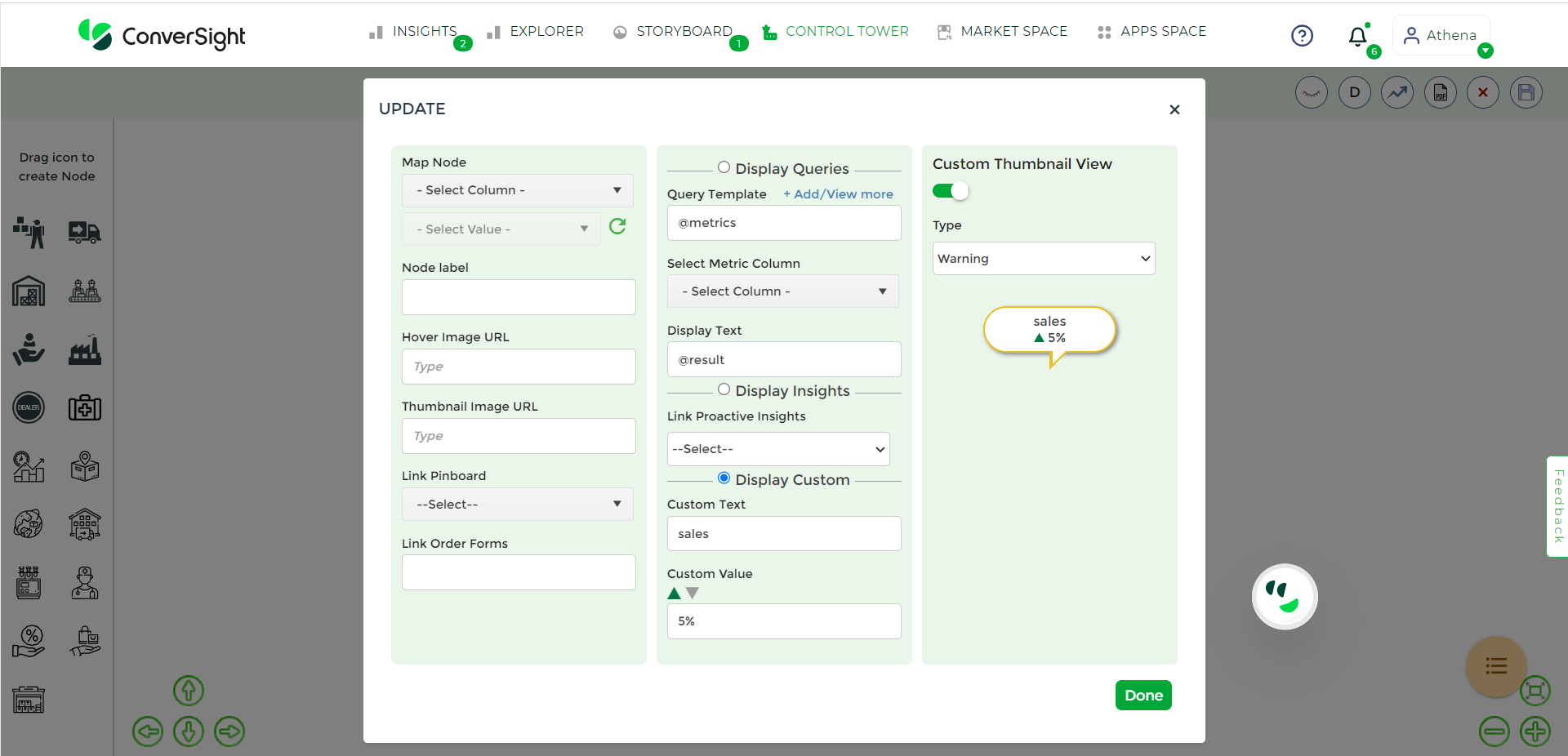
Type Warning #
Alert: Selecting the alert type will result in its display in red color.
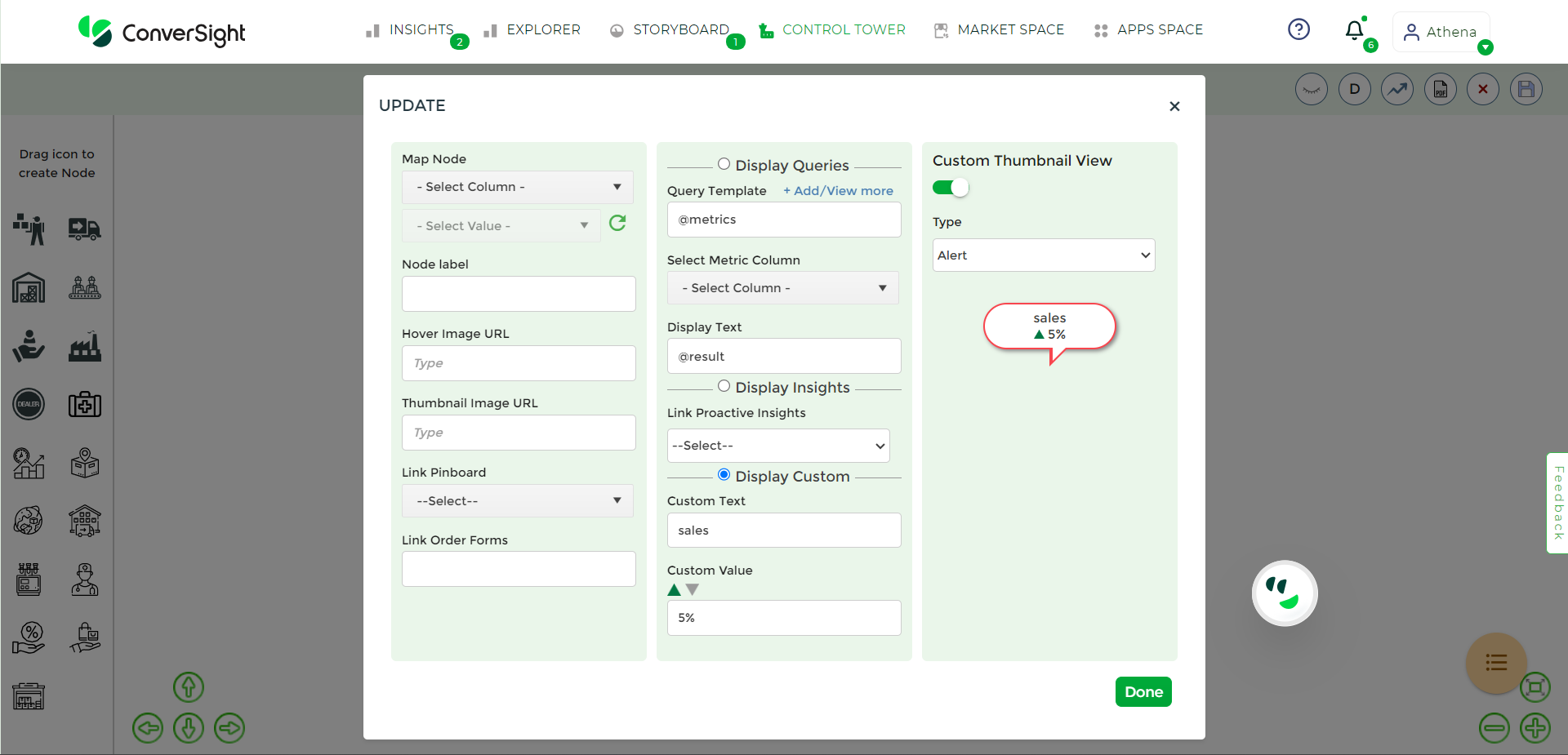
Type Alert #
After updating all the necessary columns, click the Done button to preserve the modifications.
Clone Node #
The Clone Node feature allows you to make an identical copy of a node while keeping its content and attributes intact. By right-clicking on the node you want to duplicate and choosing the Clone Node option, you can generate an exact replica of the selected node in a new instance.
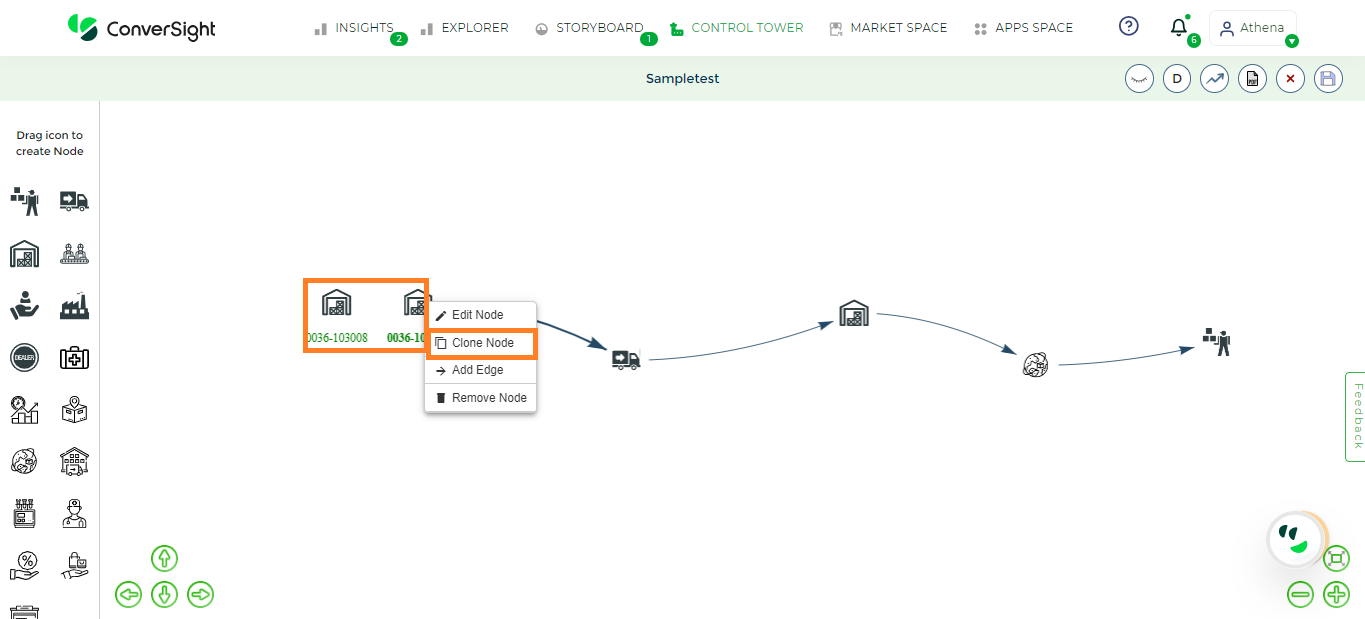
Remove Node #
The Remove Node option allows you to delete or eliminate the node that you have selected. This action results in the removal of the chosen node from the structure, effectively eliminating its presence and any associated data.
Applying filters serves to refine the information presented or analyzed by using specific criteria. Filters can be implemented within the Control Towers for each category. Upon selecting categories of dimensions or metrics, you are given the dataset’s relevant columns corresponding to the selected categories. From these columns, you can choose the ones where you wish to apply Filters.
Once you’ve chosen the categories, click on the Filter icon to implement the filters.
For applying filters to dimensions, you are provided with the selected columns, where you can choose the values presented in the columns.
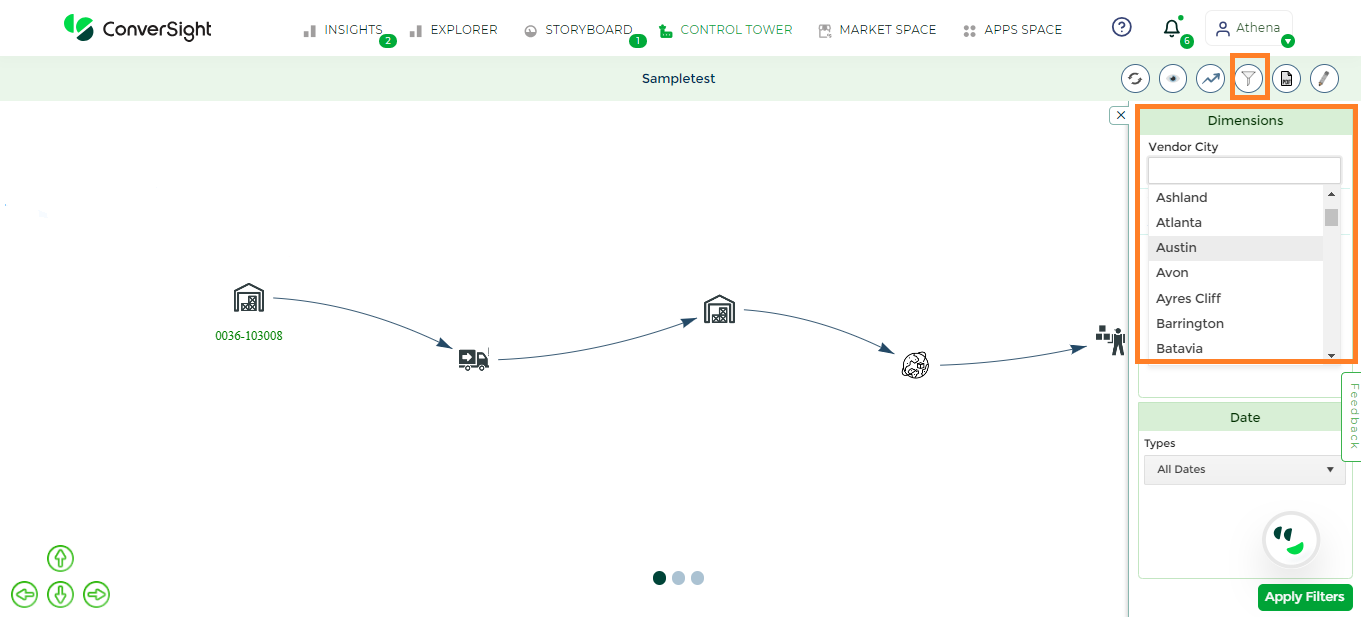
Dimensions #
For applying filters to dates, you can choose from the types that list the dates. Additionally, you can customize the dates as needed.
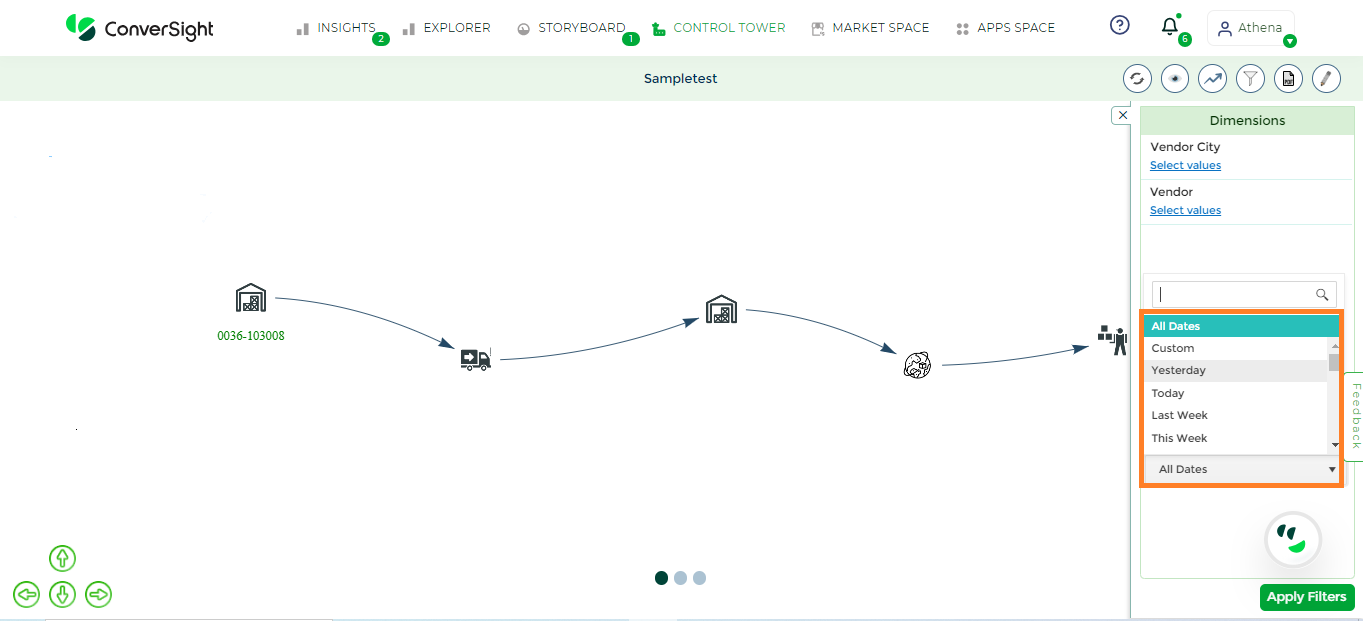
For applying filters to metrics, you’ll see the chosen metrics column listed. By clicking on the column, the filter will be applied. Clicking on Apply Filters saves the changes made to the filters and displays the results based on the applied filters.
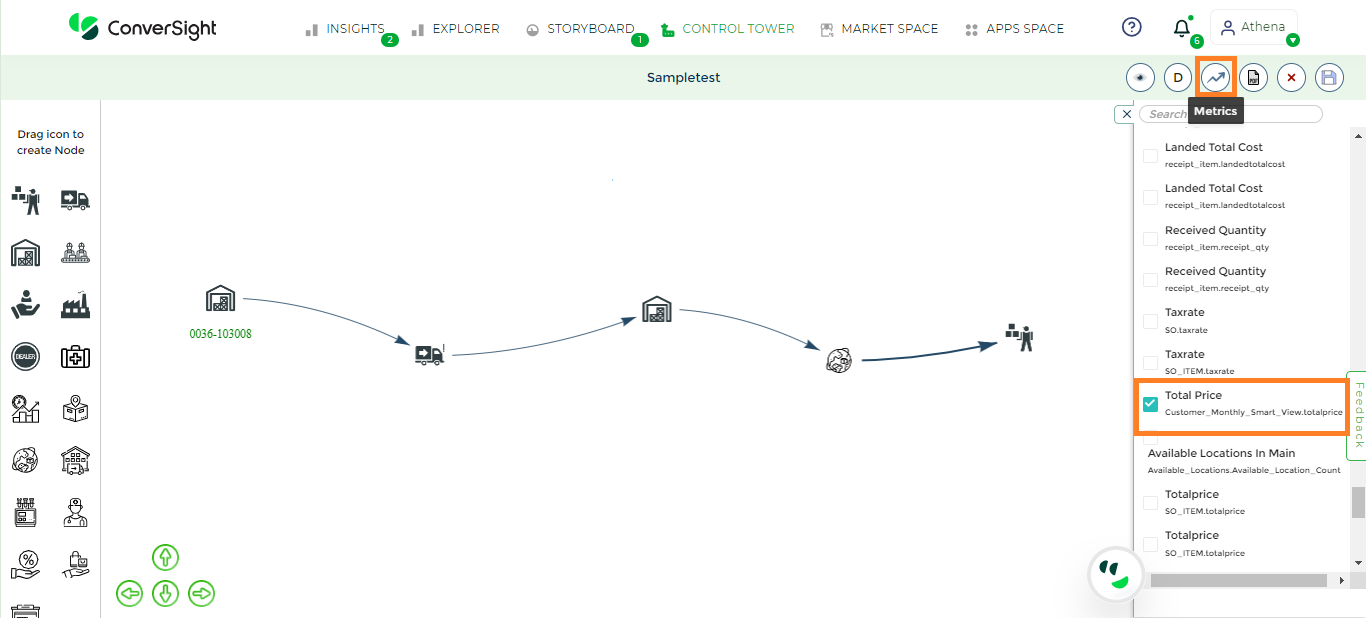
Metrics Filter #
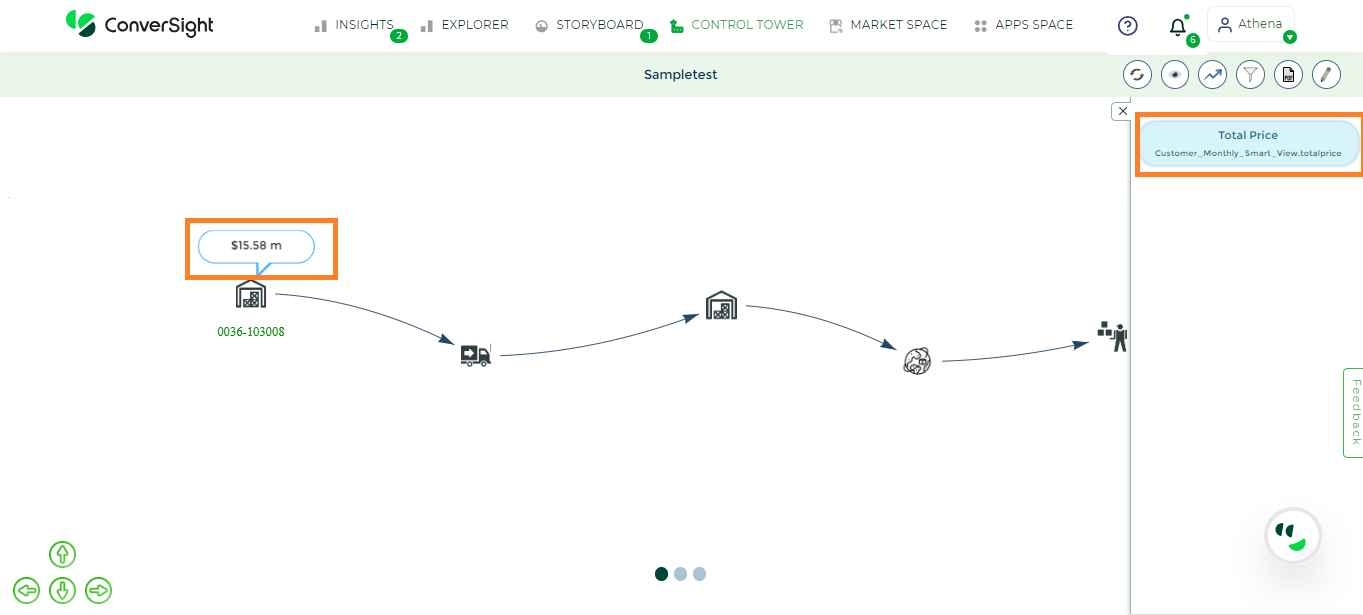
Show Legends #
Enabling the Show Legends option allows you to view the applied filters. This feature provides a clear and organized presentation of the implemented filters, offering transparency into the specific criteria used to analyze the data.
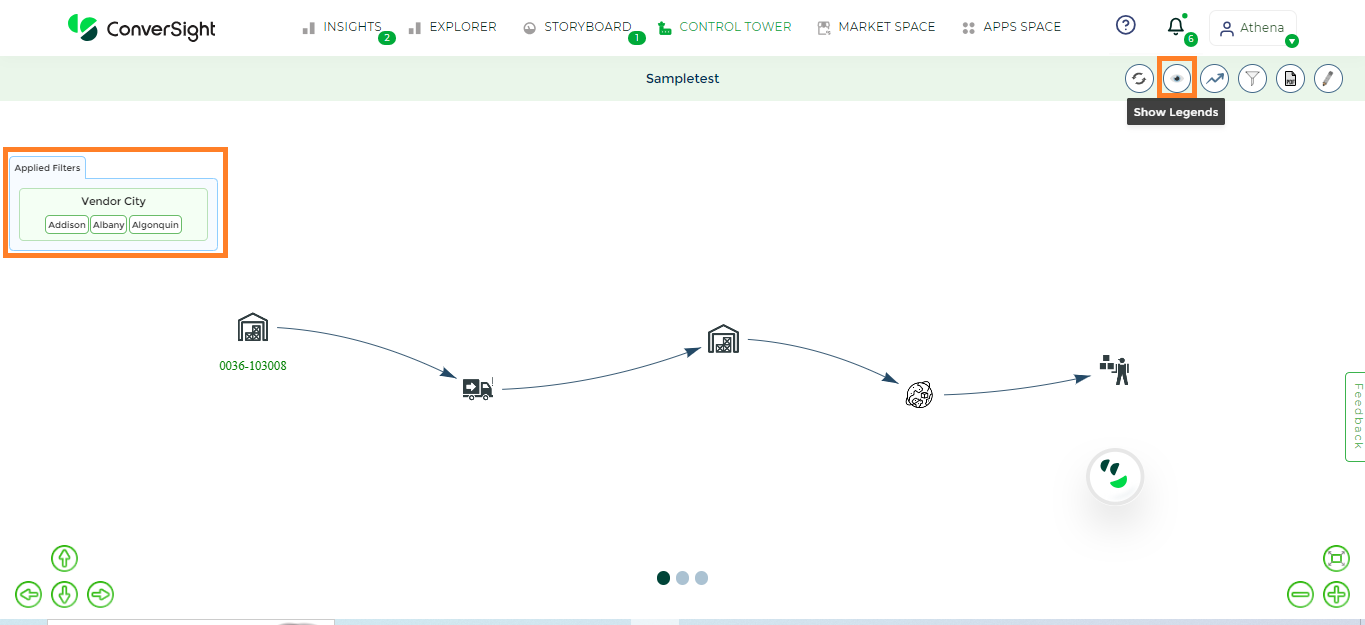
Export to PDF #
The Export to PDF option allows you to convert the reports to a static, fixed-format version, that can be easily shared, printed and viewed by others.
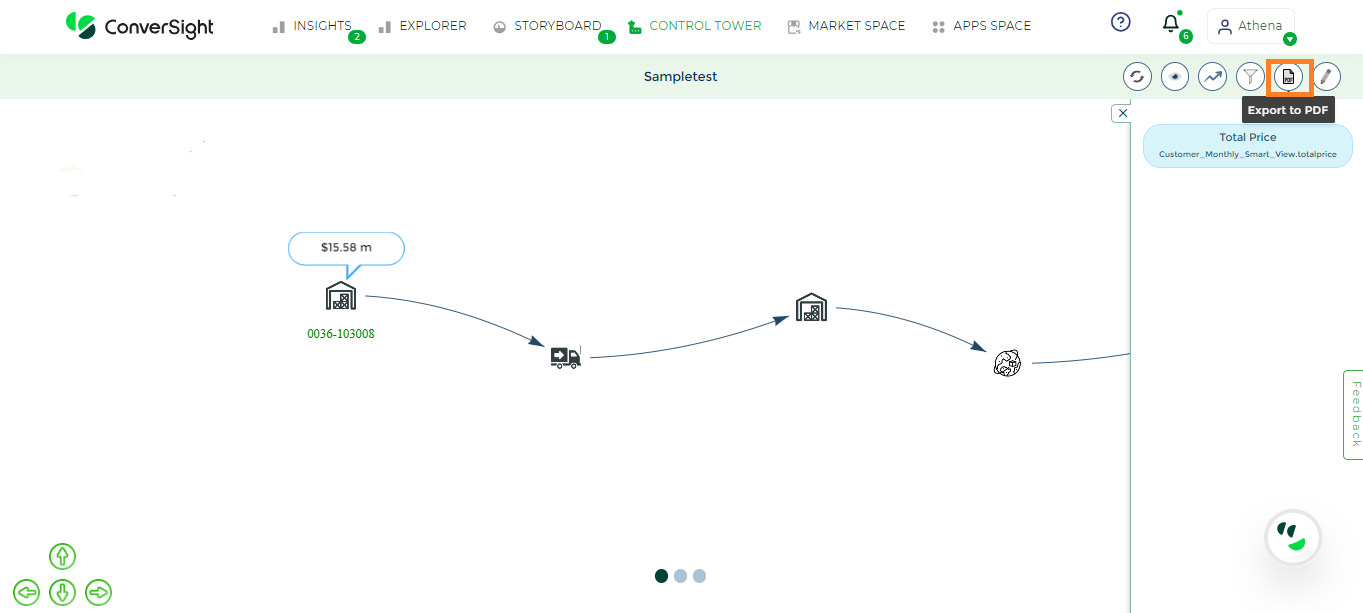
Visual Settings #
The directional arrows, including left, right, up and down, offer you the tools to modify the placement of your Control Tower, aligning it precisely with your preferred visibility. These arrows enable you to shift the Control Tower’s position, ensuring optimal observation of pertinent elements for an effective perspective.
The minus , plus and reset icons offer you precise control over adjusting your Control Towers. The minus icon reduces the size, the plus icon increases it and the reset icon restores proper alignment. Utilizing these icons allows you to fine-tune the configuration and to align your Control Towers accurately.
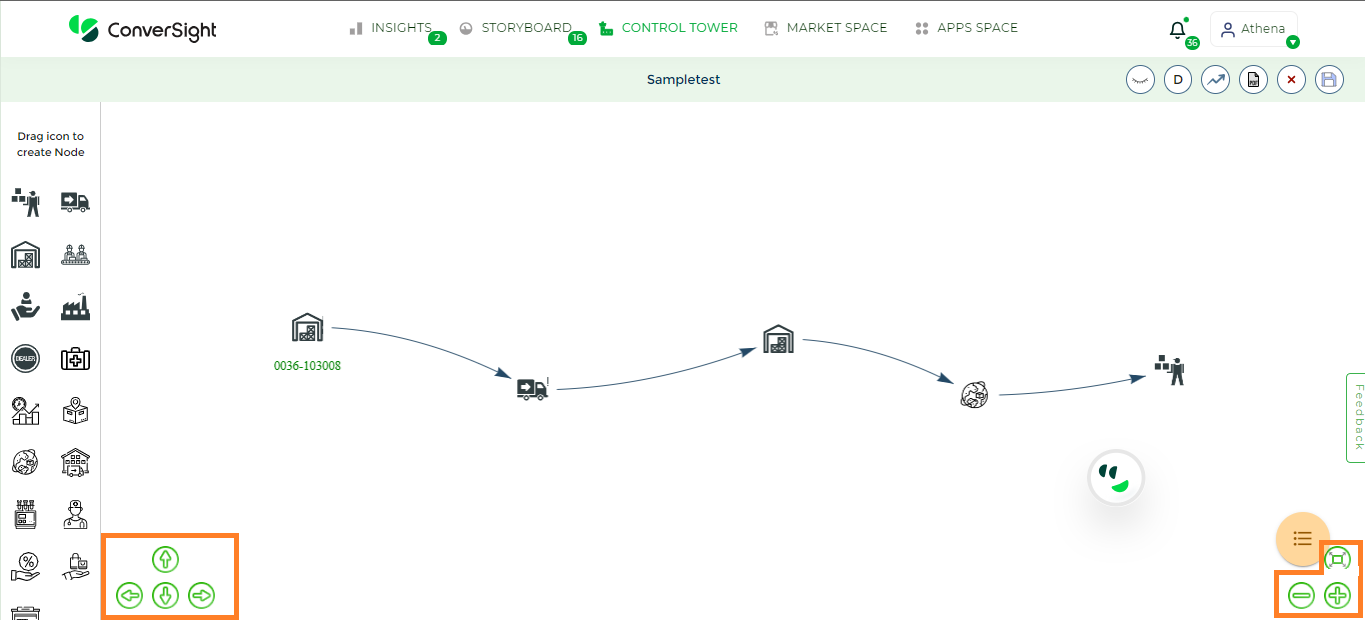
Management of Control Tower #
The Towers menu is designed to offer users a versatile array of management options, catering to various operational needs. These management capabilities encompass a range of essential actions, including:
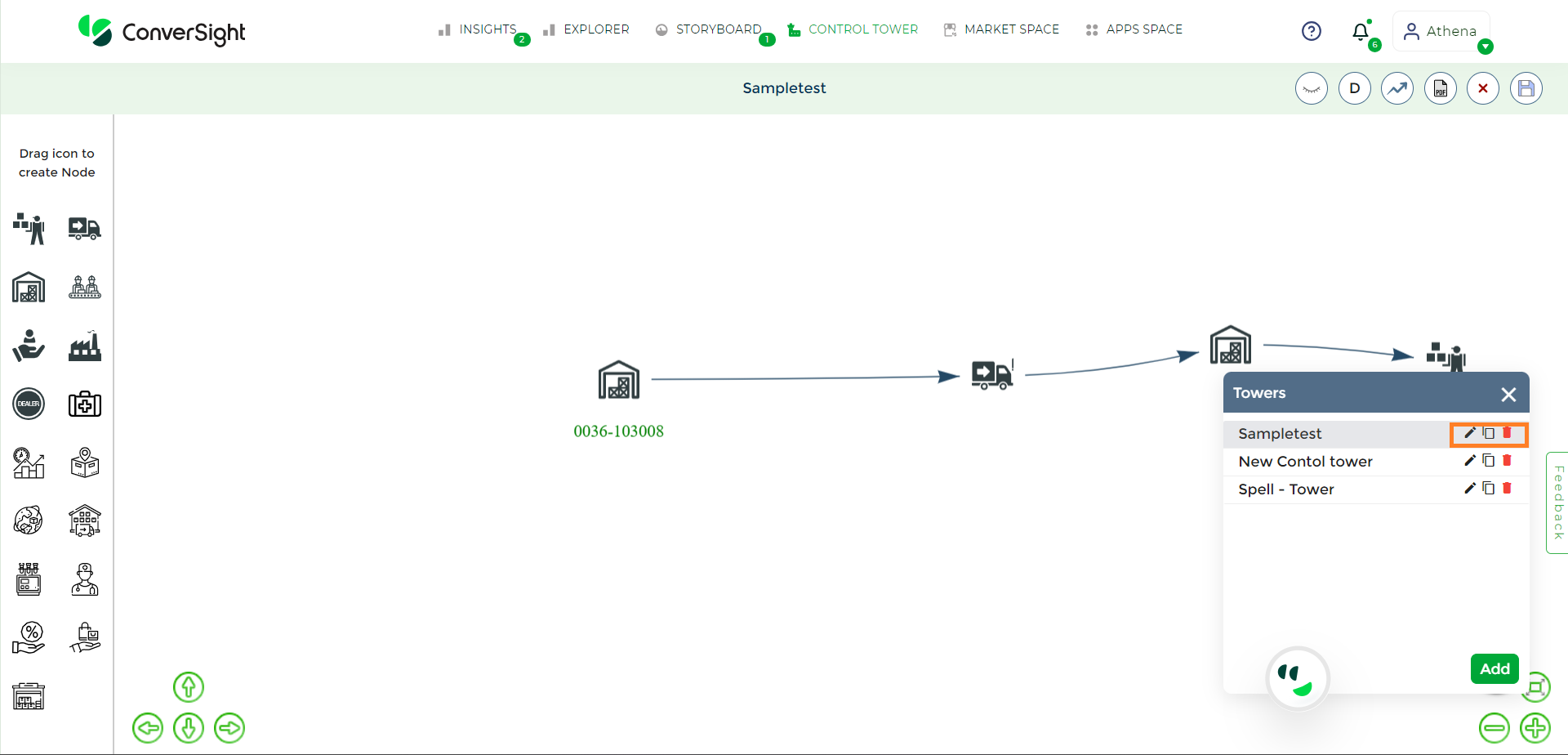
Edit Tower #
With the Edit feature, you can alter the name of the Control Tower you’ve established. However, it’s important to note that editing the dataset within the created Control Tower is not allowed. Yet, an alternative approach is that you can clone the Control Tower and then proceed to edit the dataset as needed.
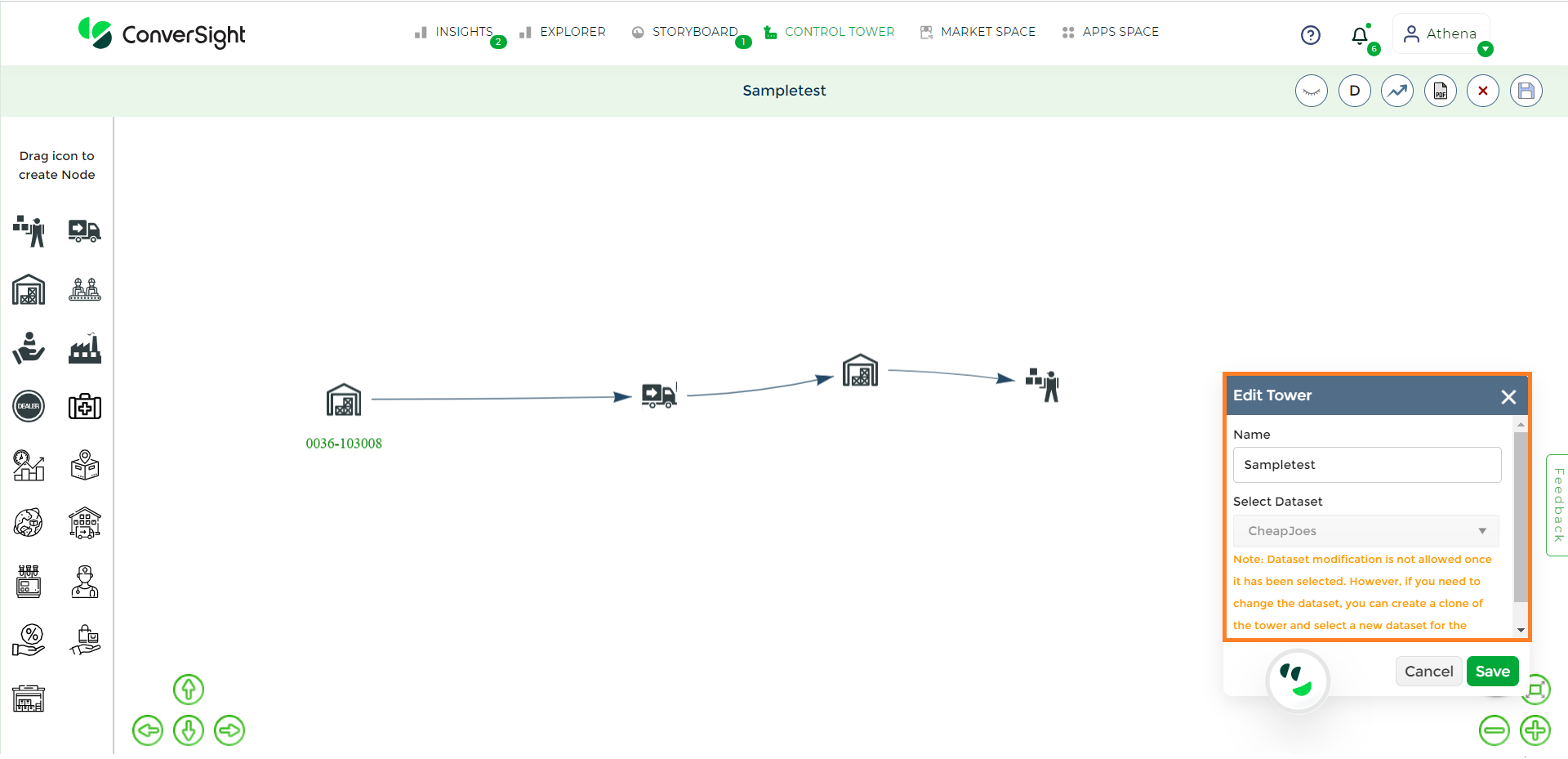
Clone Tower #
The capability of Clone Tower empowers you to create an exact replica of an existing Control Tower. The Clone option offers the subsequent choices for duplicating a Control Tower:
|
|
---|---|
| You are indeed able to create and also edit the dataset from the cloned Control Tower. |
| You can create a new Control Tower with the same dataset. |
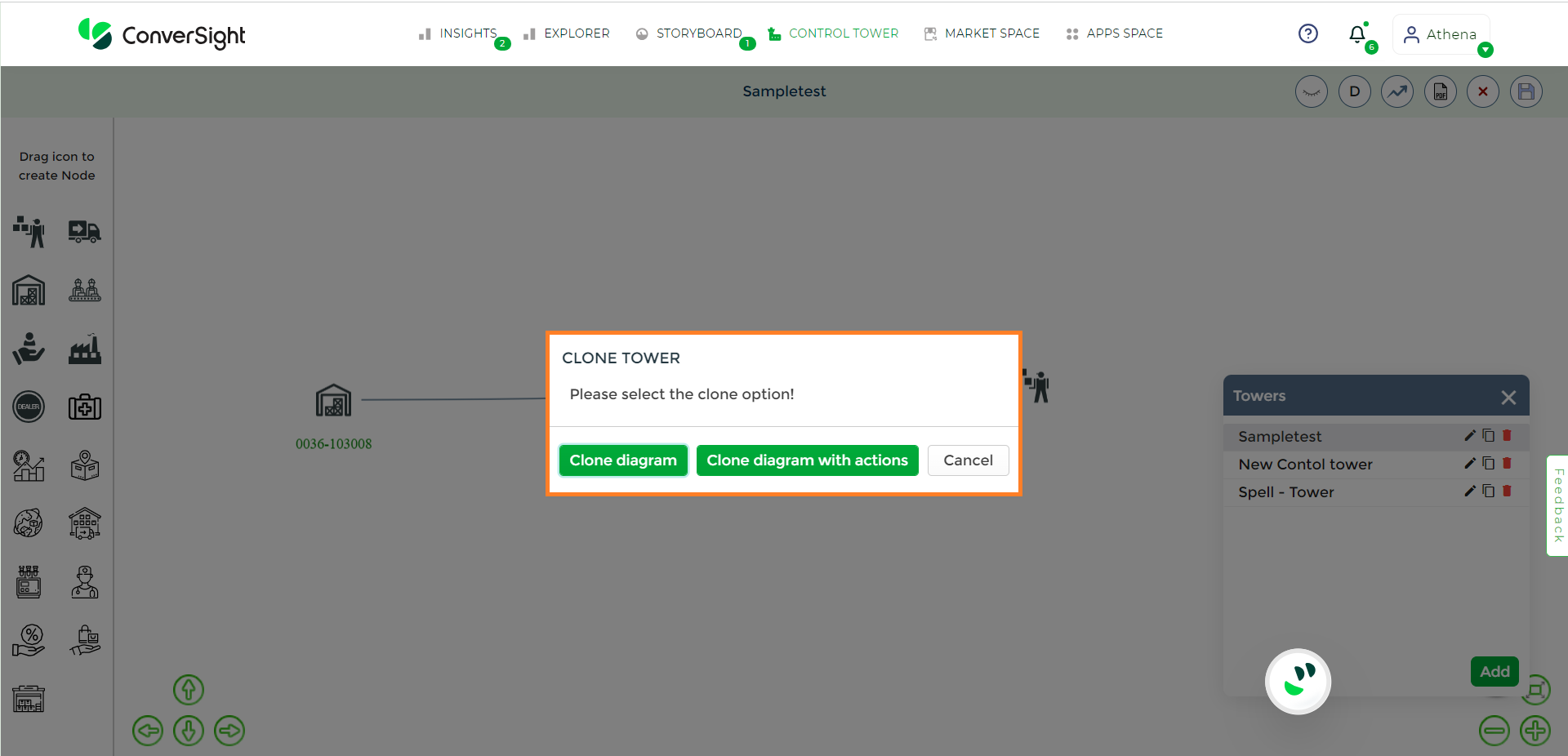
Delete Tower #
The Delete option enables the full removal of the Control Tower, including all associated data and settings, necessitating careful consideration due to its irreversible nature.
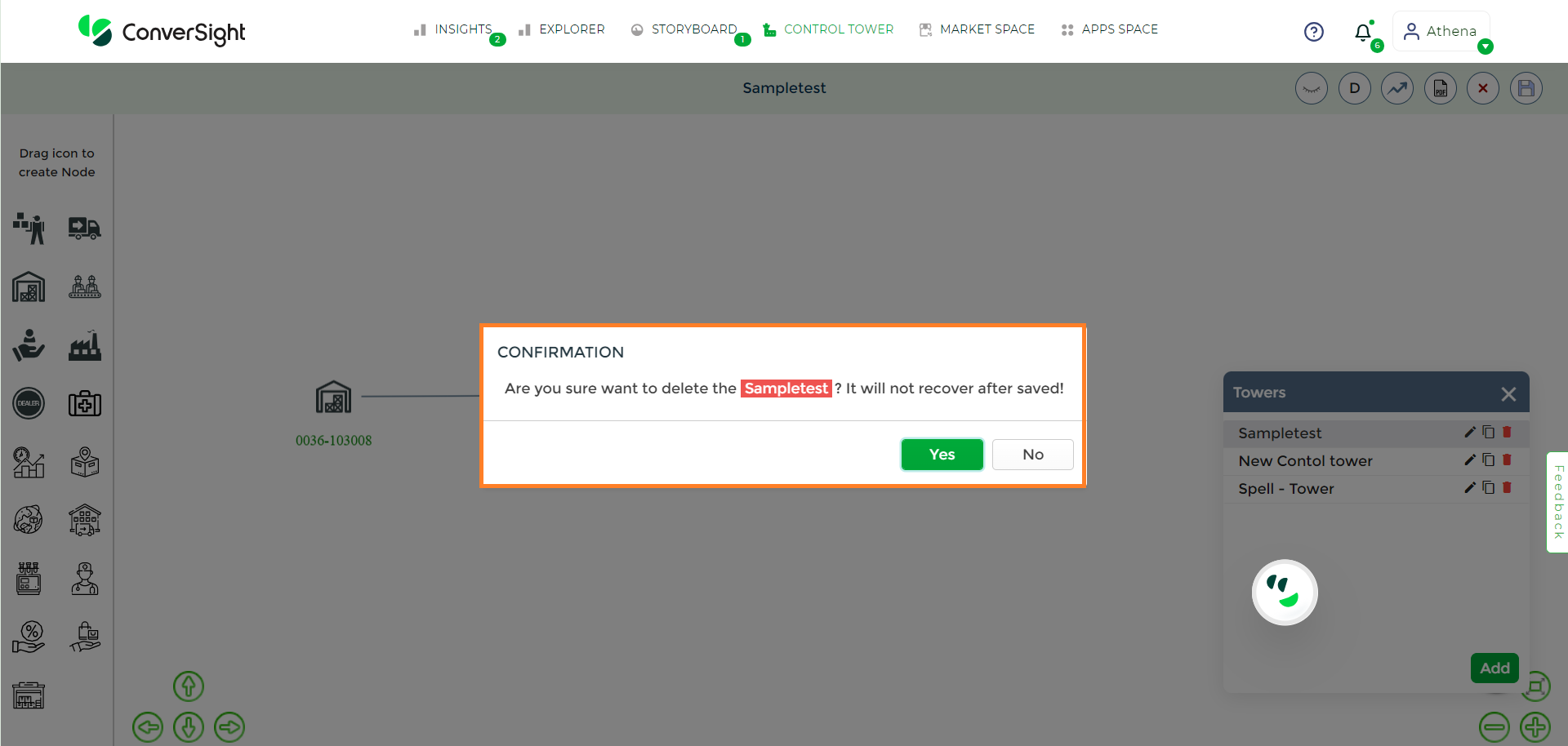
In summary, the Control Tower revolutionizes operations management by providing real-time insights and proactive response capabilities. It consolidates data for better decision-making and offers predictive insights. Its collaborative framework ensures supply chain objectives are met efficiently, while ongoing learning enriches its capabilities.

IMAGES
VIDEO
COMMENTS
Assignment 2: Control Tower. Cannot retrieve latest commit at this time. /* Assignment 2 - Control Tower */ /* Class name - must be "Assignment2" in order to run */ import java.util.Scanner; import assignment2.Airplane; import java.lang.Math.*; public class Assignment2 { public static void main (String [] args) { Scanner scan = new Scanner ...
17 votes, 17 comments. Can someone please help me. Action Movies & Series; Animated Movies & Series; Comedy Movies & Series; Crime, Mystery, & Thriller Movies & Series
Cannot retrieve latest commit at this time. /* Assignment 2 - Control Tower */ /* Class name - must be "Assignment2" in order to run */ import java.util.Scanner; import assignment2.Airplane; public class Assignment2 { public static void main (String [] args) { Airplane [] a = new Airplane [3]; a [0] = new Airplane (); a [1] = new Airplane ...
Assignment 2: Control Tower . Java I know that someone had previously posted the answer on this forum but the answer was deleted I was wondering if someone could give me the code answer Share Add a Comment. Sort by: Best ...
You will also find the assignments but you will find no answers to any of the reviews, quizzes, or exams. ... [Unit#][Lesson#][Question#].java. Unit 1: Primitive Types. Lessons 1-6. Assignment 1: Movie Ratings. Unit 2: Using Objects. Lessons 1-8. Assignment 2: Control Tower. Unit 3: Boolean Expressions and If Statements. Lessons 1-7. Assignment ...
The Control Tower assignment requires students to write a short program in Java to determine the positions of airplanes relative after changes have been made to their initial starting positions. - Assignment2_Demo.java
Question: Assignment 2: Control Tower Due No Due Date Points 10 Submitting an extemal tool Instructions In this assignment, you will be simulating an Air Traffic Control tower. This program uses data of the Airplane class type. This is a custom class that you will use for this activity. Each Airplane object represents an actual airplane that is ...
Project Stem Assignment 2 Control Tower . Java I completed it for the first two planes but I have difficulty doing the third one can someone please drop the answer? Share Sort by: Best. Open comment sort options. Best. Top. New. Controversial. Old. Q&A. Add a Comment.
Coding Activity Assignment2.java. /* Assignment 2 - Control Tower */ /* Class name - must be "Assignment2" in order to run */ import java.util.Scanner; import assignment2.Airplane; public class Assignment2 { public static void main (String [] args) { // Initialize Scanner Scanner scan = new Scanner (System.in); // User Input System.out.println ...
{"payload":{"allShortcutsEnabled":false,"fileTree":{"":{"items":[{"name":"Alternate letters if same length","path":"Alternate letters if same length","contentType ...
If you need answer for a test, assignment, quiz or other, you've come to the right place. Members Online • Impressive-Ball9623. ADMIN MOD Assignment 2: Control Tower . I need help/answers for the Assignment 2: Control Tower. Can anyone help? Thanks Share Sort by: Best. Open comment sort options. Best. Top. New. Controversial. Old. Q&A. Add a ...
Contribute to leony7/ControlTower development by creating an account on GitHub.
Computer Science questions and answers. JAVA In this assignment, you will be simulating an Air Traffic Control tower. This program uses data of the Airplane class type. This is a custom class that you will use for this activity. Each Airplane object represents an actual airplane that is detected by the tower at a particular instance in time.
If you need answer for a test, assignment, quiz or other, you've come to the right place. ... Assignment 2: Control Tower help plz Java Locked post. New comments cannot be posted. Share Sort by: Best. Open comment sort options. Best. Top. New.
You signed in with another tab or window. Reload to refresh your session. You signed out in another tab or window. Reload to refresh your session. You switched accounts on another tab or window.
Let's explore the process involved in building a Control Tower. Step 1: Navigate to the Control Tower tab in the landing page. Control Tower Tab #. Step 2: On the Control Tower page, you will encounter several choices. To initiate the creation of a new Control Tower, simply select the Edit icon located on the right-hand side.
We got you! If you need answer for a test, assignment, quiz or other, you've come to the right place. ... Members Online • Itchy_Stick_8862 . Assignment 2 Control Tower Java Can someone help? Trying to catch up on comp sci but this assignment is holding me back. Every post I find about it is outdated Locked post. New comments cannot be posted ...