Introduction
Basics of the “for-loop in golang”, golang for-loop as a “while loop”, golang for-loop as an “infinite loop”, golang for-loop as a “do while loop”, nested for-loops, for loop over channel, index as a blank identifier, value as a blank identifier, avoid using both index and value in the iteration, looping over array and slices using range for-loop, looping over maps using range for-loop, looping over strings using range for-loop, looping over integer using range for-loop.
- "Break" Loop Control Statement
- "Continue" Loop Control Statement
- "Goto" Loop Control Statement

“for-loop” vs “for-loop with range”
Time complexity, best practices, for loop in golang - a complete guide.
Golang is an excellent language and the creators of Golang have intelligently implemented for-loop in the language.
Golang is an opinionated language designed to promote simplicity, efficiency, and maintainability in software development through its specific design choices and principles.
This is the reason they have implemented “for-loop” and have ignored loops like “while”, ” do-while”, ”for each” etc.
You can achieve all these loops using only for-loops. You can try running the examples in this blog in the Online Golang playground
In Golang, a for-loop repeatedly executes a block of code based on a specified condition. The syntax of the Golang for-loop is similar to that of other languages and consists of three main components:
The initialization statement is optional; it starts its execution before the for-loop begins. It is typically a simple statement, such as a variable declaration, increment or assignment statement, or function call.
The condition statement contains a boolean expression that is evaluated at the beginning of each iteration of the loop. If the condition is true, the loop will keep executing.
Once the loop body completes its execution, the post statement is executed. Subsequently, the condition is re-evaluated. If the condition is true , the loop continues executing all the statements again. However, if the condition evaluates to false , the loop comes to an end.
The following flowchart shows how a Go for-loop works:
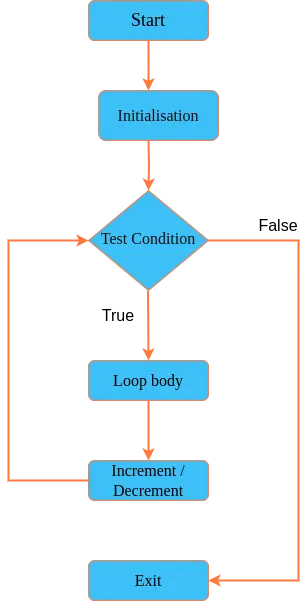
- Start : This is where the loop begins.
- Initialization Statement: This sets up the loop control variables.
- Test Condition: This checks if the loop should keep running. If it's true , the loop body runs; if it's false , the loop ends and moves to the Stop point.
- Loop Body: This is the code that runs if the condition is true .
- Update: After updating the loop control variables, the process iterates, re-evaluating the condition. If the condition is false , the loop halts.
If you skip the initialisation and update parts, a for-loop can work like a while loop. In this setup, the loop keeps going as long as the condition remains true , just like a while loop in other languages.
In the below example, the counter starts at 0. The loop continues to execute as long as the counter is less than 5. F or counter < 5 { this condition is similar to a while loop. Loop will run while the value of the counter is less than 5.
You can check out a complete example at this link
In Golang, you can make a for-loop an “Infinite Loop” by skipping the initialisation, condition, and post-statement parts. This loop will continue indefinitely until you explicitly exit it using a break statement or another control method.
In the below example, The variable winningNumber is set to 7. This is the number that will cause the loop to exit when it is randomly generated.
A for-loop is an infinite loop that runs indefinitely until a break statement is encountered.
Inside the lo op, a random number between 0 and 9 (inclusive) is generated using rand.Intn(10). if number == winningNumber condition checks if the generated number is equal to the winningNumber (which is 7).
If the condition is true, the message “You won the lottery! Exiting the loop…” is printed, and the break statement is executed to exit the loop.
Once the loop is exited, the line “Loop exited” gets printed. Enjoy your winnings!” to the console. This indicates the end of the program and celebrates the user’s “win.”
You can check out a complete example at this link
Golang does not have a do-while loop like other languages. You can simulate the behaviour of a do-while loop using a for-loop. In a do-while loop, the loop body executes at least once, followed by continued execution as long as a specified condition remains true .
We can refer to the same example as explained in the infinite loop. The for-loop structure ensures that the loop body executes at least once, similar to a do-while loop.
Nested for-loops refers to loops within other loops. They are commonly used to iterate over multidimensional data structures. Below is an example demonstrating a nested loop.
Following loop is the outer loop that iterates over the rows.
Following loop is the inner loop that iterates over the columns
Inside the inner loop, this line prints the product of i and j.
You can use a golang for-loop with a channel to send and receive data until the channel is closed. The following example illustrates sending values to a Go channel and reading those values in a Goroutine using another loop.
In the above example, the following line of code sends the value of the counter to the ch1 channel using the <- operator.
The function called channelValueReader takes a channel that contains integers as input. Inside this function, there’s a for-loop that keeps reading values from the channel using the range keyword. It continues reading values until the channel is closed. Each time it gets a value, it prints out “Current value in the channel is: ” followed by the value itself.
For loop with range keyword / Foreach loop
Range is used to iterate over various data structures like slices, maps, arrays, and channels. It returns two values: the first is the index as an integer value, and the second is the value copy of the element at that index. The range keyword in Go functions similarly to a “for each” loop found in other programming languages. Following is the basic syntax for range.
Blank identifier
A blank identifier is used to ignore the returned values that you don’t need. You can ignore the index, ignore the value, or ignore both.
In the following example, the for-loop iterates over each element of the colour slice. It uses the range keyword to get both the index and value of each element. However, the index is assigned to the blank identifier _, indicating that we want to ignore it.
In the following example, the for-loop iterates over each element of the colour slice. The index of each element is assigned to the variable index, while the value of each element is assigned to a blank identifier, indicating that we want to ignore it. Even if you don’t mention “_” here, it will also give a return value as an index.
In the following example The for-loop iterates over each element of the color slice. Here both the index and value are ignored.
It returns the first value as the index of the current element, and the second value is the value of the current element. You can check out a complete example at this link
The first value that is returned by the map is the key, and the second value returns the value from the key-value pair. You can check out a complete example at this link
Using range on the string, it returns the first value as index, and the second value is rune(int32). You can check out a complete example at this link
In Go version 1.22, a new feature has been introduced that allows you to use the for-loop with the range keyword to iterate over integers. You can find more information about this feature in the Go 1.22 release notes on the official Go blog
Loop Control Statements
"break" loop control statement.
The “break” statement stops the loop before it would normally end based on its condition.
In this example, the loop iterates through a list of signals. If the signal is red, it breaks the loop and prints the signal and its index. You can check out a complete example at this link
"Continue" Loop Control Statement
It skips the current iteration of the loop and proceeds with the next iteration.
In the above example, the loop iterates through a list of signals. If the signal is green, it continues to the next iteration, printing that it is continuing at the green signal. If the signal is not green, it prints that it is stopping at the signal and exits the loop. You can check out a complete example at this link
"Goto" Loop Control Statement
It starts jumping to a labelled statement elsewhere in the code.. You can check out a complete example at this link
for-loop with range
Determine by the number of iterations and the operations within the loop. Hence, for basic loops, it is O(n) , and for nested loops, it is O(n*m) , where n represents the number of iterations for the outer loop and m represents the number of iterations for the inner loop.
- Use clear and descriptive names for your variables so others can easily understand your code.
- Keep the processing inside loops to a minimum to make your code faster and easier to understand.
- Choose the right data structures for your needs to make your code run efficiently.
- Avoid using nested loops when you can to make your code simpler and faster.
Priyanka Chavan

We ❤️ Golang
- 1. On-Demand Golang Developers / Team
- 2. Software Development Outsourcing
Popular Tutorials
Popular examples, certification courses.
Created with over a decade of experience and thousands of feedback.
Go Introduction
- Getting Started with Go
- Go Variables
- Go Data Types
- Go Print Statement
- Go Take Input
- Go Comments
- Go Operators
- Go Type Casting

Go Flow Control
- Go Boolean Expression
- Go if...else
Go for Loop
Go while Loop
Go break and continue
Go Data Structures
- Go Functions
- Go Variable Scope
Go Recursion
- Go Anonymous Function
- Go Packages
Go Pointers & Interface
- Go Pointers
- Go Pointers and Functions
- Go Pointers to Struct
- Go Interface
- Go Empty Interface
- Go Type Assertions
Go Additional Topics
- Go defer, panic, and recover
Go Tutorials
- Golang Type Assertions
In programming, a loop is used to repeat a block of code. For example,
If we want to print a statement 100 times, instead of writing the same print statement 100 times, we can use a loop to execute the same code 100 times.
This is just a simple example; we use for loops to clean and simplify complex programs.
- Golang for loop
In Golang, we use the for loop to repeat a block of code until the specified condition is met.
Here's the syntax of the for loop in Golang.
- The initialization initializes and/or declares variables and is executed only once.
- Then, the condition is evaluated. If the condition is true , the body of the for loop is executed.
- The update updates the value of initialization .
- The condition is evaluated again. The process continues until the condition is false .
- If the condition is false , the for loop ends.
- Working of for loop
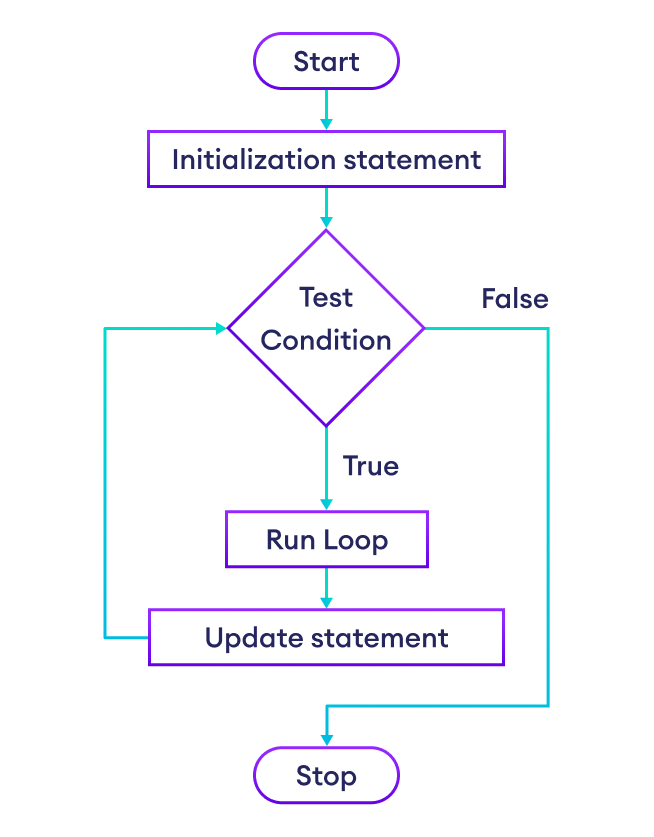
Example 1: Golang for loop
Here is how this program works.
Example 2: Golang for loop
Here, we have used a for loop to iterate from i equal to 1 to 10.
In each iteration of the loop, we have added the value of i to the sum variable.
Related Golang for Loop Topics
In Go, we can use range with for loop to iterate over an array. For example,
Here, we have used range to iterate 5 times (the size of the array). The value of the item is 11 in the first iteration, 22 in the second iteration, and so on. To learn more about arrays, visit Golang Arrays
In Golang, for loop can also be used as a while loop (like in other languages). For example,
Here, the for loop only contains the test condition . And, the loop gets executed until the condition is true . When the condition is false , the loop terminates.
A loop that never terminates is called an infinite loop. If the test condition of a loop never evaluates to true, the loop continues forever. For example,
Here, the condition i <= 10 never evaluates to false resulting in an infinite loop.
If we want to create an infinite loop intentionally, Golang has a relatively simpler syntax for it. Let's take the same example above.
In Golang, we have to use every variable that we declare inside the body of the for loop. If not used, it throws an error. We use a blank identifier _ to avoid this error.
Let's understand it with the following scenario.
Here, we get an error message index declared but not used .
To avoid this, we put _ in the first place to indicate that we don't need the first component of the array( index ). Let's correct the above example with a blank identifier.
Here, the program knows that the item indicates the second part of the array.
Table of Contents
- Introduction
- Example: Golang for loop
- Example: Sum of Natural Numbers
Sorry about that.
Our premium learning platform, created with over a decade of experience and thousands of feedbacks .
Learn and improve your coding skills like never before.
- Interactive Courses
- Certificates
- 2000+ Challenges
Related Tutorials
Programming
